No tutorial anterior, aprendemos sobre a sintaxe do Python — ou seja, seus identificadores, palavras-chave, comentários, instruções, literais e tipos de dados. Também aprendemos que Python é orientado a objetos e que esses objetos podem ser mutável ou imutável. Objetos Python que apontam para um grupo de valores são iterável, que, neste caso, é chamado de iterador.
Todos os tipos de dados abordados no tutorial anterior (inteiros, flutuantes, números complexos, strings, strings brutas) são tipos de dados para literais (valores reais atribuídos a variáveis ou atributos). Python também possui tipos de dados para referências que apontam para um grupo de valores (itens) ordenados ou não ordenados.
Além disso, Python possui os seguintes tipos de dados:
Tuplas – uma sequência de objetos. Uma sequência refere-se a um grupo ordenado de itens (objetos), onde os índices podem acessar os itens. Os itens de uma tupla podem ser um objeto arbitrário mutável ou imutável.
No entanto, a tupla é imutável, o que significa que os itens de uma tupla não podem ser atualizados ou alterados depois de definidos. É por isso que raramente é recomendado usar itens mutáveis em uma tupla, a menos que eles nunca sejam alterados ou exijam atualização. Uma tupla pode ser vista como um grupo de valores ou objetos constantes e pode ser usada para definir uma ou mais constantes.
Uma tupla é definida como um grupo de valores ou objetos separados por espaços ou vírgulas. O grupo pode ser opcionalmente colocado entre parênteses (“(“). As vírgulas também são opcionais, mas é recomendado usar vírgulas para separar itens da tupla, principalmente se a tupla for necessária para uma função.
Opcionalmente, o último item da tupla também pode ter uma vírgula no final. Uma tupla vazia é definida por um par vazio de parênteses. A função integrada, tupla , também pode ser usado para definir uma tupla. Qualquer grupo de itens separados por espaços ou vírgulas será padronizado como uma tupla. Se uma única string (ou qualquer iterável) for definida como uma tupla, seus caracteres (ou itens) poderão ser usados como itens de tupla.
Aqui estão alguns exemplos válidos de tuplas:
tup1 = 'Id' 'Nome' 'Endereço'
tup1 = 'Id', 'Nome', 'Endereço'
tup1 = ('Id', 'Nome', 'Endereço')
tup1 = ('Id', 'Nome', 'Endereço',)
tup1 = #Tupla Vazia
tup1 = ('Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro)
tup1 = ('Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro,)
tup1 = tupla('Id', 'Nome', 'Endereço')
tup1 = tupla('Id' 'Nome' 'Endereço')
tup1 = tuple('ABC') # é equivalente a tup = ('A', 'B', 'C')
Listas – sequências ordenadas como um grupo de itens mutáveis. Isso significa que os itens de uma lista podem ser alterados ou modificados. Essencialmente, as listas são um grupo modificável de valores ou objetos onde os itens do grupo podem ser substituídos, removidos ou adicionados. Os itens de uma lista podem incluir qualquer objeto arbitrário mutável ou imutável.
Uma lista é definida como um grupo de valores e/ou objetos separados por vírgulas entre colchetes (“(“). Opcionalmente, o último item da lista pode ter uma vírgula final. A função integrada, listatambém pode ser usado para definir uma lista.
Uma lista vazia é definida por um par de colchetes vazios ou pelo lista função sem argumentos. Se um iterável for usado como único item de uma lista, seus itens poderão ser usados como itens da lista.
Aqui estão alguns exemplos válidos de listas:
list1 = ('Id', 'Nome', 'Endereço')
list1 = ('Id', 'Nome', 'Endereço',)
lista1 = #Lista Vazia
lista1 = lista #Lista Vazia
lista1 = ('Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro)
lista1 = ('Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro,)
lista1 = lista('Id', 'Nome', 'Endereço')
lista1 = lista('Id' 'Nome' 'Endereço')
lista1 = lista('ABC') # é equivalente a lista1 = ('A', 'B', 'C')
Conjuntos – uma coleção mutável e não ordenada de itens exclusivos. Os itens de um conjunto devem ser imutáveis e “capazes de hash”, o que significa que seu valor (o valor hash) nunca deve mudar. Imutável significa que seu tipo de dados também nunca deve mudar. Isso ocorre porque um conjunto só pode conter itens imutáveis e capazes de hash, sem qualquer outro conjunto dentro dele.
Um conjunto é definido por um grupo de valores e/ou objetos (imutáveis e hasháveis) separados por vírgulas entre colchetes (“{“). Opcionalmente, o último item do conjunto pode ter uma vírgula final. A função integrada, definir, também pode ser usado para definir o conjunto. Um conjunto vazio é definido por um par vazio de colchetes ou definir função sem argumentos.
Aqui estão exemplos válidos de conjuntos:
set1 = {'Id', 'Nome', 'Endereço'}
set1 = {'Id', 'Nome', 'Endereço',}
set1 = {} #Conjunto vazio
set1 = set #Conjunto Vazio
set1 = {'Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro}
set1 = {'Id', 123, 0b1111_1110, 2.5j5.4, Verdadeiro,}
set1 = set('Id', 'Nome', 'Endereço')
set1 = set('Id' 'Nome' 'Endereço')
Conjuntos congelados – versão imutável de conjuntos. Eles são definidos pelo conjunto congelado função. Um frozenset vazio é definido pelo conjunto congelado função sem argumentos.
Aqui estão exemplos válidos:
frset1 = frozenset('Id', 'Nome', 'Endereço')
frset1 = frozenset('Id' 'Nome' 'Endereço')
frset1 = frozenset #Empty Conjunto Congelado
Dicionário – uma coleção mutável e não ordenada de valores emparelhados com chaves arbitrárias. Essas chaves são arbitrárias, mas devem ser capazes de hash. Os valores podem incluir quaisquer objetos mutáveis ou imutáveis arbitrários.
O dicionário é semelhante a um mapa não ordenado ou a uma tabela hash em outras linguagens de programação. É definido como um grupo de vírgulas, pares separados de chaves e valores entre colchetes ou usados como argumentos no ditado função.
O par chave-valor, chave e valor são separados por dois pontos (“:”). No ditado função, chave e valor em um par podem ser separados por um sinal de igual (“=”) ou podem ser colocados em uma tupla. Opcionalmente, o último item (par chave-valor) de um dicionário pode ter uma vírgula final.
Um dicionário vazio é definido por um par vazio de colchetes ou pelo ditado função sem argumentos. Qualquer item em um dicionário deve ser um par de valores-chave, mesmo que o valor seja iterável.
Aqui estão exemplos válidos de dicionários:
ditado1 = {x:25, y:57, z:64}
ditado1 = {x:25, y:57, z:64,}
ditado1 = {'x':25, 'y':57, 'z':64,}
dict1 = {'x':25, 57:'y', z:8.9j6.5}
ditado1 = ditado (x = 25, y = 57, z = 64)
dict1 = dict((x, 25), (y, 57), (z, 64))
dict1 = dict # Dicionário vazio
dict1 = {} # Dicionário vazio
Nenhum – um objeto nulo em Python, que é equivalente a “nenhum objeto” e possui zero atributos ou métodos. Uma função retorna Nenhum se não tiver uma instrução de retorno.
Byte – sequências imutáveis (coleção ordenada), onde apenas itens podem ser bytes (inteiros no intervalo de 0 a 255). Os itens de um Byte podem ser caracteres de uma string, números inteiros, Nenhumou iteráveis.
Bytes podem ser definidos pré-fixando “b” antes de uma string ou inteiro ou usando um byte função. A função Byte também permite especificar uma codificação.
Aqui estão alguns exemplos válidos:
Val = b'Python'
Val = b'123'
Val = bytes('Python')
Val = bytes('Python', utd-8)
Val = bytes ((1, 2, 3, 4, 5))
Matrizes de bytes – sequências mutáveis (coleção ordenada), contendo apenas itens (inteiros no intervalo de 0 a 255). Os itens de uma matriz de bytes podem ser caracteres de uma string, inteiros, Nenhumou iteráveis.
A única diferença entre Byte e Matriz de bytes tipos é que um byte é imutável, enquanto a matriz de bytes é mutável. Uma matriz de bytes pode ser definida usando o recurso integrado bytearray função.
Aqui estão alguns exemplos válidos:
Val = bytearray(“Python”, 'utf-8')
Val = bytearray((1, 2, 3, 4, 5,))
Val = bytearray # bytearray vazio
Faixa – uma sequência (coleção ordenada) de números inteiros entre dois valores inteiros, de modo que cada valor inteiro (item) difere por um item adjacente por meio de um valor de etapa. Por padrão, os valores adjacentes são separados por uma diferença de “1”.
Uma gama é refinada usando o recurso integrado faixa função. A função range tem a seguinte sintaxe:
intervalo(iniciar, parar, passo)
- Se apenas um valor for fornecido como argumento no range , ele fornecerá uma sequência de inteiros de 0 até o argumento fornecido.
- Se dois valores forem fornecidos como argumentos, será fornecida uma sequência de números inteiros entre os dois valores, que os inclui.
- Se três valores forem fornecidos como argumentos, o terceiro valor servirá como a diferença entre cada item adjacente.
Aqui estão exemplos válidos de um intervalo:
intervalo(9) # 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
intervalo(5, 10) # 5, 6, 7, 8, 9, 10
intervalo(2, 10, 2) #2, 4, 6, 8, 10
Boleano – este tipo pode ter apenas dois valores: Verdadeiro ou Falso. Qualquer valor diferente de zero ou contêiner não vazio é considerado True. Qualquer valor zero, Nenhumou Vazio contêiner, é considerado falso. Os valores Verdadeiro e Falso também podem ser denotados por “1” e “0”, respectivamente.
Existem vários outros tipos de dados em Python que podem ter classes integradas (como data e hora) ou classes definidas pelo usuário. Por exemplo, qualquer classe que defina um tipo de dados por finalidade ou denote uma estrutura de dados pode ser considerada uma classe de tipo de dados.
Existem seis tipos de sequências (coleções ordenadas) em Python:
- Cordas
- Bytes
- Matrizes de bytes
- Faixa
- Tuplas
- Listas
Os índices das sequências devem começar em “0” (ou seja, o primeiro item de qualquer sequência tem um índice/chave “0”). conjuntos, conjuntos congelados e dicionários são coleções não ordenadas em Python.
Para maior clareza, consulte esta tabela:
Nome da coleção | Natureza da Coleção | Natureza dos Itens |
Corda | Sequência imutável (coleção ordenada) de caracteres ASCII ou UTF (ou outra codificação) | Caracteres ASCII ou UTF ou caracteres correspondentes ao esquema de codificação aplicado |
Byte | Sequência imutável de bytes (0 a 255) | Valores de bytes no intervalo de 0 a 255 que podem ser inteiros, caracteres ou iteráveis |
Matriz de Bytes | Sequência mutável de bytes | Valores de bytes no intervalo de 0 a 255 que podem ser inteiros, caracteres ou iteráveis |
Faixa | Sequência imutável de inteiros | Números inteiros entre o intervalo definido |
Tupla | Sequência imutável de objetos | Objetos arbitrários mutáveis ou imutáveis |
Lista | Sequência mutável de objetos | Objetos arbitrários mutáveis ou imutáveis |
Definir | Coleção mutável e não ordenada de objetos | Objetos imutáveis arbitrários, objetos duplicados não permitidos |
Conjunto Congelado | Coleção imutável e não ordenada de objetos | Objetos imutáveis arbitrários, objetos duplicados não permitidos |
Dicionário | Coleção mutável e não ordenada de pares de valores-chave | Chaves imutáveis arbitrárias e valores mutáveis ou imutáveis arbitrários |
Variáveis, atributos e itens
Em Python – onde tudo é um objeto – os valores dos dados também são objetos. Os valores dos dados são armazenados na memória. Isso significa que sempre que um novo valor de dados aparece no código Python (seja definido no código ou em tempo de execução), ele também é armazenado em algum local da memória.
O código acessa esses valores de dados por meio de referências. Essas referências podem ser variáveis, atributos, métodos ou itens de um objeto. As variáveis são referências a valores de dados que podem ser usados em qualquer lugar do código.
Os atributos são referências a valores de dados não solicitáveis associados a um objeto. Métodos são referências a valores de dados “chamáveis” associados a um objeto. Ser chamável significa que esses valores de dados executam um grupo de instruções em sua ocorrência. Em Python, as instâncias de uma classe são chamadas de itens. Portanto, itens são referências a instâncias de uma classe ou objetos.
Sempre que um valor de dados é atribuído a uma variável, atributo, método ou objeto, o processo é chamado de ligação. O valor dos dados permanece independente de sua ligação. Se um valor de dados diferente for atribuído a uma referência, esse valor de dados será armazenado em um local de memória diferente. Como resultado, o valor dos dados anteriores (se não estiver vinculado a qualquer outra referência) torna-se desvinculado. A limpeza de valores ou objetos de dados não vinculados é chamada de coleta de lixo.
Não há declarações em Python. As referências aparecem instantaneamente e não possuem tipo de dados declarado. A forma como uma referência (a um valor/objeto de dados) é tratada depende da expressão em que ela aparece e como/se é usada para religar outra referência.
Os valores dos dados podem ser vinculados a uma referência por meio de uma atribuição ou de instruções de atribuição aumentada. Em uma instrução de atribuição, uma expressão RHS é vinculada a um destino LHS e eles são separados pelo sinal de atribuição (“=”). O destino pode ser qualquer referência, como uma variável, um atributo ou um item.
Uma declaração de atribuição simples se parece com isto:
var = Expressão
Este é um exemplo de tarefa simples:
uma = 1
É possível atribuir um valor de dados (ou o resultado de uma expressão) a vários destinos ao mesmo tempo:
var1 = var2 = var3 = Expressão
Este é um exemplo de tal tarefa:
uma = b = c = 1
Se a expressão RHS for iterável ou resultar em iterável, ela deverá ser atribuída a outro iterável (do mesmo tamanho) ou a múltiplas variáveis (igual em número ao tamanho do iterável) da seguinte forma:
var1, var2, var3 = Iterável/Expressão resultando em Iterável
Aqui está um exemplo de tal afirmação:
a, b, c, = (1,1, 1,9, abc)
Se uma referência de destino tiver um asterisco (“*”) antes dela, ela aceitará uma lista de todos os valores/objetos de dados que não foram atribuídos, como segue:
var1, *var2, var3 = Iterável/Expressão resultando em Iterável
Aqui está um exemplo de tal tarefa:
a, *b, c, = 1,1, 1,9, abc, 43, 2,5j4 # b = 1,9, abc, 43
Em uma atribuição aumentada, um operador aumentado, como +=, -=, *=, **=, é usado para uma atribuição. A referência de destino deve ser criada antes do uso em tal instrução. Só pode haver uma referência de destino em uma atribuição aumentada.
Na atribuição aumentada, o valor de dados atribuído anteriormente a uma referência é modificado e religado a ela. O valor de dados atribuído anteriormente, então, torna-se desvinculado (daquela referência específica).
Aqui está um exemplo de tarefa aumentada:
uma += b # uma = uma + b
Os valores dos dados podem ser desvinculados das referências usando um del declaração. Isso é chamado de desvinculação. É possível desvincular múltiplas referências em um único del instrução separando as referências de destino por uma vírgula. No entanto, um del A instrução apenas desvincula um valor/objeto de dados de uma referência e não exclui o valor ou objeto de dados. Se o valor dos dados não estiver vinculado a qualquer outra referência, ele será deixado para coleta de lixo.
Aqui estão exemplos válidos de del declarações:
del a
del a, b, c
Operadores Python
Os operadores são utilizados para manipular valores de dados e com referências formando as expressões lógicas. Pode haver uma ou mais expressões dentro de uma instrução lógica. Python oferece suporte a muitos operadores.
Estes são os mais comuns:
Operadores aritméticos – incluem adição (+), subtração (-), multiplicação
, divisão (/), módulo (%), exponenciação (**) e divisão de piso (//). O operador de adição também serve como operador de concatenação com strings e outros iteráveis. Da mesma forma, o operador de multiplicação
pode ser usado para copiar uma string ou iterável pelos tempos especificados usando um multiplicador. Operadores de atribuição –
incluem atribuir (=), adicionar e atribuir (“+=”), subtrair e atribuir (-=), multiplicar e atribuir (*=), dividir e atribuir (/=), módulo e atribuir (%=), exponenciação e atribuir (**=) e divisão de piso e atribuir (//==).
Operadores de comparação –
incluem igual (==), diferente (!= ou ), maior que (>), menor que (<), maior ou igual a (>=) e menor ou igual a (<=). Também é possível encadear comparações da seguinte forma:
a < b >= c # a < b e b >=c Operadores lógicos –
incluem AND lógico (e), OR lógico (ou) e NOT lógico (não). Operadores bit a bit –
incluem AND binário (&), OR binário ( ), XOR binário (^), complemento binário (~), deslocamento binário para a esquerda (<<) e deslocamento binário para a direita (>>). Operadores de adesão – incluir em (em) e não em (não em). Esses operadores são usados para avaliar se um item pertence a uma sequência ou coleção. O operador in (in) retorna verdadeiro se o item LHS for membro da sequência/coleção RHS. O operador not in (not in) retorna verdadeiro se o item LHS não for membro da sequência/coleção RHS. Operadores de identidade – include é (é) e não é (não é). Esses operadores são usados para avaliar se duas referências são idênticas. As duas referências podem ter a mesma identidade se apontarem para o mesmo valor de dados, que pode ser armazenado no mesmo local de memória (dependendo do sistema operacional e da plataforma). O é
O operador (is) retorna verdadeiro se ambas as referências tiverem a mesma identidade. O não é
O operador (não é) retorna verdadeiro se ambas as referências não tiverem a mesma identidade. Operador de indexação –
o operador de indexação ( ) é usado com sequências para acessar um item específico delas. Os índices em todas as sequências começam com “0”, de modo que o primeiro item de qualquer sequência tenha um índice (ou chave) de 0. Os índices dos itens subsequentes aumentam em “1”. Portanto, x(2) permite acessar o terceiro item de uma sequência “x”. Se um número negativo for utilizado como índice, esse item será acessado contando a partir do último item da sequência e não do primeiro.
Operador de fatiamento –
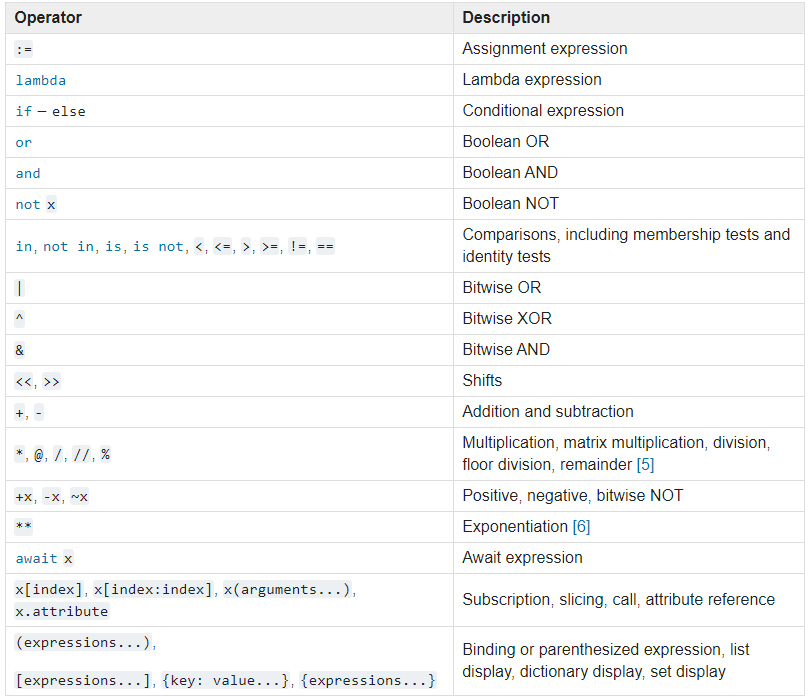
Precedência do operador em Python
A tabela a seguir resume a precedência do operador em Python, da precedência mais baixa à precedência mais alta:
Precedência de operadores em Python
Tabela mostrando precedência de operadores em Python em ordem crescente Instruções de fluxo de controle
O Python oferece suporte às seguintes instruções de fluxo de controle:
If-elif-else –
uma instrução condicional que permite executar instruções se as expressões condicionais retornarem verdadeiras. Tem esta sintaxe:
se expressão_condicional1:
declarações)
elif expressão_condicional2:
declarações)
elif expressão_condicional3:
declarações) senão expressão_condicional: declarações) No se declaração, o Elif e outro
são opcionais. Como uma expressão condicional é avaliada como True, as instruções que a precedem são executadas e a execução do
se
declaração termina aí. No entanto, uma expressão condicional não precisa retornar explicitamente como True ou False. Em Python, qualquer expressão ou objeto avalia implicitamente True ou False como uma expressão condicional.
Portanto, recomenda-se usar a seguinte expressão:
Se x:
Se não x:
Em vez das seguintes expressões:
Se x == Verdadeiro:
Se x == 1:
Se x for verdadeiro:
Se bool(x):
Se x == Falso: Se x == 0: Se x for falso:
É importante notar que Python faz não
apoiar uma instrução switch.
Enquanto declaração –
repete uma instrução ou bloco de instruções se uma expressão condicional for avaliada como True. Ele continua repetindo até que a expressão condicional seja avaliada como False. Isso significa que uma instrução deve conter código que eventualmente torne a expressão condicional Falsa.
A instrução while tem esta sintaxe: Enquanto expressão_condicional: Declarações) As instruções que são executadas repetidamente em um enquanto instrução, são chamados de corpo de loop. Se o enquanto é usado dentro de uma função, e o corpo do loop contém uma instrução return, o loop termina aí – pois a instrução return encerra a execução da função. Da mesma forma, o quebrar e continuar
declarações também podem afetar o enquanto declaração. Declaração para entrada – permite executar uma instrução ou bloco de instruções enquanto itera através de itens de um iterável. Uma referência de destino acessa os itens do iterável. Os itens do iterável podem ser usados pela(s) instrução(ões) ou não. Mas o iterável nunca deve ser modificado em um para dentro
declaração como a
enquanto
iterando através de uma lista, conjunto ou dicionário, os itens não devem ser adicionados ou removidos deles.
A instrução for-in tem esta sintaxe: para target_reference em iterável: Declarações)
Pode haver mais de uma referência de destino separada por vírgulas se o iterável for um dicionário ou outra estrutura de dados em que cada item contenha mais de um valor de dados. Por exemplo, o seguinte
para
instrução itera através de um dicionário: para chave, valor em d.items : imprimir(chave + ' : ' + valor + '/n')
Se uma única instrução tiver que ser executada em cada item de um iterável para obter um novo iterável, o
para
instrução pode ser combinada com uma instrução de atribuição da seguinte forma:
y = x+3 para x no intervalo(5)
Nessa instrução, a execução da expressão também pode ser condicional, assim: y = x+3 para x no intervalo(5) se x%2==0 Declarações semelhantes são possíveis com listas, conjuntos e dicionários. Declaração de quebra –
usado em um corpo de loop para encerrar o loop. Muitas vezes é usado com um
se
declaração. À medida que a instrução break é encontrada, o loop termina.
Aqui estão alguns exemplos válidos de uma instrução break:
Enquanto x: #execute loop se x não for zero ou nenhum
Se x<10:
imprimir (x)
x + = 1
outro:
quebrar
Enquanto x: #execute loop se x não for zero ou nenhum
Se x>10:
quebrar
outro: imprimir (x) x + = 1 Continuar declaração –
usado em um corpo de loop para deixar a execução de instruções depois dele apenas para a iteração atual do loop. Muitas vezes é usado com o
se
declaração.
Aqui está um exemplo válido:
enquanto x<=10: se x%2 == 0: continuar imprimir (x)Outra declaração – usado com umse , para e enquanto declarações. Quando usado com opara ou enquanto ele executa uma instrução ou bloco de instrução após o término natural do loop (ou seja, após todos os itens de um iterável no para
declaração ou quando uma expressão condicional de um enquanto declaração é avaliada como Falsa). Declaração de aprovação –
usado no
se
declaração se nada deve ser feito para uma expressão condicional. Tem esta sintaxe:
se expressão_condicional:
Declarações)
elif expressão_condicional:
Declarações)
elif expressão_condicional:
Passe #Nada a fazer se esta condição for atendida outro: Declarações)Manipulação de exceção – Suporte para Pythontentar ,exceto ,finalmente , outro e com
instruções para tratamento de exceções. Uma exceção pode ser levantada no código explicitamente por um
elevação
- declaração. Quando uma exceção é levantada implícita ou explicitamente, a execução normal do código se desvia até que a exceção seja resolvida.
- Funções
- Funções são blocos de instruções que podem ser chamados. Em Python, funções também são objetos. Eles podem ser:
Atribuído a referências (variáveis, atributos e outros objetos) Incluído como um item em um iterável Usado como argumento em outras funções
Uma função também pode retornar outra função. É definido usando um
definição
instrução e tem esta sintaxe:
def nome_função(parâmetros):
declarações)
O nome da função é um identificador que pode ser usado para fazer chamadas de função. Os parâmetros são referências que devem ser fornecidas a uma função quando ela é chamada. Os parâmetros (ou argumentos) são opcionais e se uma função não requer o fornecimento de referências, não há razão para incluí-los na definição da função.
Se houver vários parâmetros, eles deverão ser separados por vírgulas entre parênteses na definição da função. Pode haver uma ou mais instruções executadas ao chamar a função. Essas instruções são chamadas de corpo da função.
É possível ter parâmetros opcionais em uma função que podem ou não ser fornecidos quando a função é chamada. Esses parâmetros opcionais são especificados por uma expressão de atribuição, onde o parâmetro opcional recebe um valor padrão.
Uma função com parâmetros opcionais possui esta sintaxe:
def nome_função(parâmetro, parâmetro_opcional = expressão):
declarações)
Além disso, este é um exemplo válido de função com parâmetros opcionais:
def makeList(x, y= ): y.append(x) retornar
Uma função pode retornar qualquer valor de dados ou objeto usando um
retornar
instrução, que só pode ser usada no corpo de uma função. Se a função não tiver uma instrução return, uma expressão, um valor de dados ou um objeto após uma palavra-chave return, a função não retornará nenhum objeto.
Os parâmetros que não são opcionais são chamados de parâmetros posicionais. É possível fornecer qualquer número arbitrário de parâmetros posicionais para uma função com um asterisco pré-fixado
ou asterisco duplo (**) antes de um parâmetro.
Ao pré-fixar um asterisco
a um parâmetro, ele aceita qualquer número arbitrário de parâmetros posicionais e os coleta em uma tupla. Ao pré-fixar um asterisco duplo
a um parâmetro, ele aceita qualquer número arbitrário de parâmetros posicionais e os coleta no dicionário.
Aqui está um exemplo válido de aceitação de um número arbitrário de parâmetros posicionais em uma função:
def adicionar(*num)
soma de retorno (num)
s = adicionar(15, 20, 15) # s = 50
As referências fornecidas a uma função quando chamada são conhecidas como argumentos. Os argumentos podem ser fornecidos como argumentos posicionais ou nomeados. Se os valores/objetos de dados forem usados como argumentos sem serem atribuídos a referências de parâmetros, eles serão aceitos na chamada de função pela posição de sua aparição na assinatura da função. (Nota: a assinatura da função é a coleção de parâmetros vinculados a uma função).
Estes são chamados de argumentos posicionais. Se os valores/objetos de dados forem usados como objetos, atribuindo-os explicitamente a referências de parâmetros, a posição de sua aparência em uma chamada de função não importa. Eles são chamados de argumentos nomeados ou de palavras-chave.
Aqui está um exemplo válido dos argumentos posicionais:
def f(a, b, c):
retornar a = b = c
f(12, 17, 21) # retorna 50
Este é um exemplo válido de argumentos nomeados (palavra-chave):
def f(a, b, c):
retornar a = b = c
f(b = 12, a = 17, c = 21) # retorna 50
Em Python é possível definir uma função dentro de outra função e esta é chamada. A função definida dentro de outra função é chamada de função aninhada e a função na qual outra função é definida é chamada de função externa. Uma função aninhada pode usar os parâmetros e referências de uma função externa. Essa função aninhada é chamada de encerramento.
Uma função também pode retornar os valores/objetos em momentos diferentes. Por exemplo, pode ter um rendimento ou rendimento de declarações. Tais funções são chamadas de geradores. Quando um gerador é chamado, ele retorna o valor/objeto ou resultado de uma expressão na primeira instrução de rendimento e salva todas as referências locais em sua memória.
Quando a função é chamada novamente, ela inicia a execução a partir das instruções após a primeira instrução de rendimento e retorna o valor/objeto ou resultado da expressão após outra instrução de rendimento. O rendimento de uma instrução pode ser usado se a expressão que o contém for um iterador.
Aqui está um exemplo válido de uma função geradora:
def f(a, b, c):
produzir um
rendimento a + b
rendimento a + b + c
para x em f(2, 5, 7): print(x) # retorna 2, 7, 14
O gerador usa o rendimento das instruções:
def f(a, b, c):
rendimento do intervalo (a)
rendimento do intervalo (b)
rendimento do intervalo (c)
para x em f(1, 2, 3): print(x) # retorna 0, 1, 0, 1, 2, 0, 1, 2, 3
Python também suporta funções recursivas, que são aquelas que se autodenominam dentro do próprio corpo.
Aqui está um exemplo válido de função recursiva em Python: def fatorial (n): se n == 1: retorne 1
senão retorne n * fatorial (n-1)
Escopo de referências
Em Python, as referências podem ter escopo local ou global. Isso é chamado de namespace. As referências que se ligam a uma função ou a outros objetos são locais para essa função ou objeto. Essas referências podem ser usadas somente dentro da função ou objeto ao qual estão vinculadas.
As referências que aparecem fora das funções ou de outros objetos têm escopo global. Essas referências podem ser usadas em qualquer lugar do código. Uma referência pode ser tornada global prefixando um
global
palavra-chave. Este é um exemplo válido:uma = 1
deff(b):
global bretornar a + bNopróximo tutorial , aprenderemos mais sobre matrizes de bytes, listas, conjuntos e dicionários. Embora strings, bytes, intervalo e tupla sejam sequências imutáveis, a matriz de bytes e a lista são apenas sequências mutáveis em Python. Conjuntos e coleções são usados com mais frequência em coleções não ordenadas.