Um dos principais atrativos da plataforma Raspberry Pi é o seu módulo de câmera. Atualmente, a Raspberry Pi Foundation oferece três módulos de câmera:
• Módulo de Câmera 2
• Módulo de câmera 2 NoIR
• Câmera Raspberry Pi de alta qualidade
O módulo de câmera 2 é um substituto para o módulo de câmera original em abril de 2016. O módulo V2 possui um sensor Sony IMX219 de 8 megapixels em comparação com o sensor OmniVision OV5647 de 5 megapixels no módulo original. O módulo é compatível com Raspberry Pi 1, 2, 3 e 4. Ele pode ser facilmente conectado à interface serial da câmera (porta CSI) de qualquer Raspberry Pi. O módulo de câmera V2 suporta modos de vídeo 1080p30, 720p60 e VGA90. O novo módulo não é apenas de alta resolução. É muito melhor em qualidade de imagem, desempenho em pouca luz e fidelidade de cores. O módulo de câmera infravermelha 2 é igual ao módulo normal 2, exceto que não utiliza um filtro infravermelho. A câmera Pi NoIR é muito útil para fotografia noturna e captura de vídeo. A câmera de alta qualidade é um sensor Sony IMX477 de 12,3 megapixels que suporta lentes de montagem C e CS.
Você pode desenvolver muitos projetos interessantes com o módulo de câmera Raspberry Pi. Bem, apenas instalando um módulo de câmera em seu minúsculo computador de bolso, você pode explorar o vasto mundo do processamento de imagem, processamento de vídeo e até mesmo aprendizado de máquina. Neste artigo, exploramos como começar a usar o módulo de câmera e controlá-lo usando Python.
O que você precisa
Tudo que você precisa é de um Raspberry Pi (1/2/3/4) e do módulo da câmera. O módulo de câmera padrão é usado para tirar fotos sob luz. A câmera NoIR Pi tira fotos no escuro e requer uma fonte de luz infravermelha adicional. Neste artigo, estamos trabalhando com o módulo de câmera padrão. O módulo da câmera deve vir com um cabo flexível para conexão à porta CSI. Para conectar a câmera ao Raspberry Pi Zero, é necessário um cabo menor.
Como conectar o módulo da câmera
Todos os Raspberry Pi possuem uma interface serial de câmera (porta CSI). O módulo da câmera é conectado à porta CSI. É comum confundir a porta CSI com a porta display. A localização da porta CSI no Raspberry Pi é indicada abaixo.
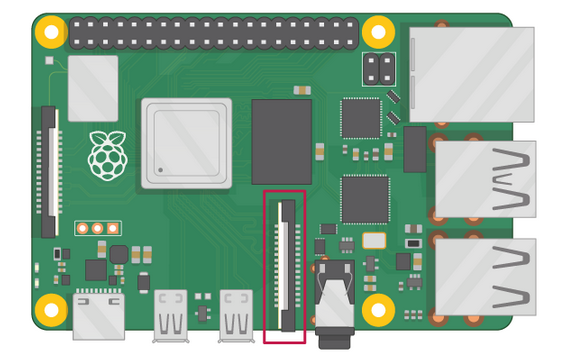
Exemplo de módulo de câmera Raspberry Pi 2
Para instalar o módulo da câmera, localize a porta CSI. Remova com cuidado o clipe plástico da porta. Insira o cabo plano do módulo da câmera na porta CSI de forma que os conectores na parte inferior do cabo fiquem voltados para os contatos da porta. Coloque o clipe de plástico de volta para que o cabo plano fique preso. O módulo da câmera conectado ao Raspberry Pi se parece com o mostrado abaixo.
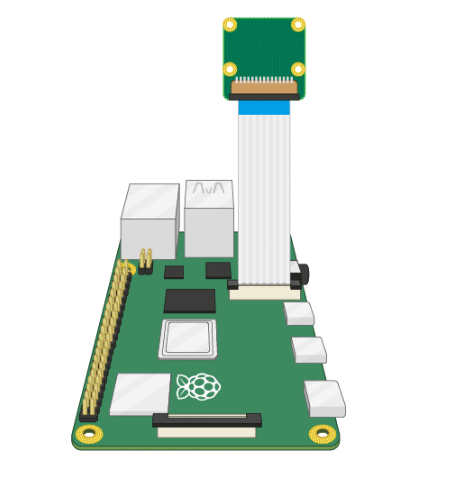
Posição da porta CSI no Raspberry Pi
Ativar câmera Raspberry Pi
O módulo da câmera não está habilitado por padrão. Após conectar o módulo da câmera, inicialize o Raspberry Pi. Vá ao menu principal e abra a ferramenta de configuração do Raspberry Pi. Você também pode abrir a ferramenta de configuração no terminal digitando sudo raspi-config.
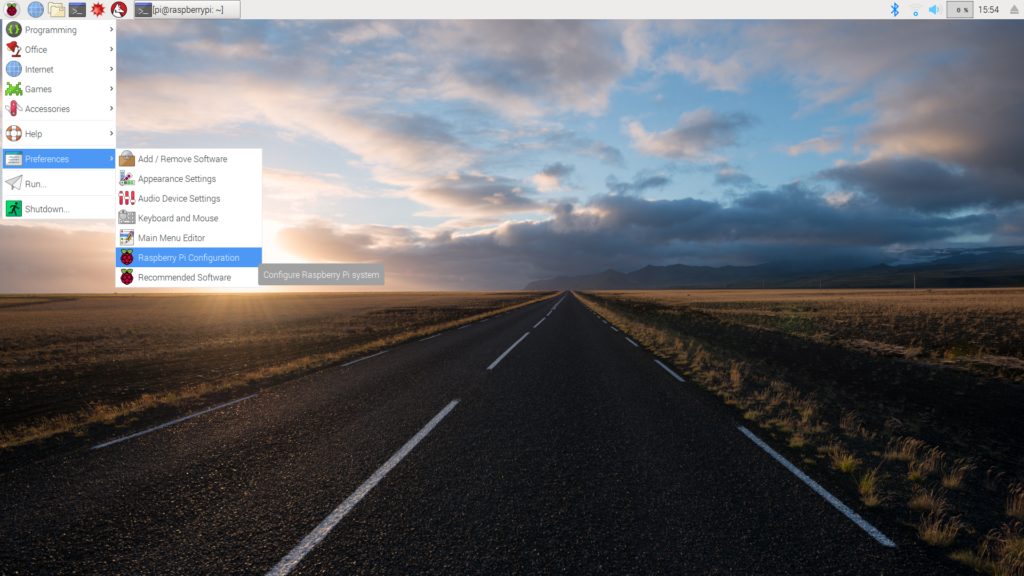
Instalando a câmera Raspberry Pi
Na ferramenta de configuração, selecione a aba interfaces e certifique-se de que a câmera esteja habilitada.
Se não estiver habilitado, habilite a câmera e reinicie o Raspberry Pi.
Tirando fotos da linha de comando
Depois que o módulo da câmera estiver instalado e habilitado, é hora de testá-lo. O melhor lugar para testar o módulo da câmera é a linha de comando do Raspberry Pi, ou seja, o shell Bash. Abra a janela do terminal clicando no ícone do terminal na barra de tarefas.
Para tirar uma foto, digite o seguinte comando e pressione Enter.
raspistill -o Desktop/image.jpg
O comando acima tira uma foto usando o módulo da câmera e a salva na área de trabalho. A visualização da câmera abre por cinco segundos quando o comando acima é executado e fecha quando uma imagem estática é capturada. As imagens podem ser salvas em qualquer outro local, desde que a pasta especificada exista na estrutura de diretórios do Raspberry Pi.
Capturando um vídeo da linha de comando
Para gravar um vídeo usando o módulo da câmera, digite o seguinte comando e pressione Enter.
raspivid -o Desktop/video.h264
O comando acima captura uma gravação de vídeo e salva o vídeo gravado em uma pasta especificada quando interrompido. Assim como as imagens, os vídeos também podem ser salvos em qualquer local, desde que a pasta especificada exista na estrutura de diretórios do Raspberry Pi. Os vídeos salvos podem ser reproduzidos usando um player VLC.
Controlando o módulo da câmera com Python
O módulo da câmera pode ser controlado usando Python. Isso requer a biblioteca picamera. No Raspberry Pi 3/4, a biblioteca picamera já vem instalada com o IDLE ou Thonny Python IDE. Se a biblioteca picamera estiver faltando, ela poderá ser instalada para todos os usuários usando os seguintes comandos.
$ sudo apt-get install python-picamera
Lembre-se de atualizar a instalação após instalar a biblioteca picamera usando os seguintes comandos.
$ sudo apt-get atualização
$ sudo apt-get atualização
A biblioteca picamera também pode ser instalada para todos os usuários que usam PIP.
$ pip instalar picamera
Em um script Python, a biblioteca picamera pode ser importada usando os comandos a seguir.
da importação de picamera PiCamera
Para usar o módulo câmera em um script Python, um objeto da classe picamera precisa ser instanciado da seguinte maneira.
câmera = PiCâmera
Testando o módulo da câmera com Python
Para testar o módulo da câmera com Python, a melhor maneira é executar a visualização da câmera por algum tempo. Se o módulo da câmera estiver instalado corretamente e funcionando com a placa Raspberry Pi, um LED vermelho piscará no módulo da câmera sempre que a placa Raspberry Pi for inicializada. O LED vermelho permanece aceso quando a câmera está ativa.
Salve o seguinte script Python em um arquivo .py e execute o código para ver a visualização da câmera.
da importação de picamera PiCamera
do tempo importar sono
câmera = PiCâmera
câmera.start_preview
dormir (5)
câmera.stop_preview
Deve-se observar que o arquivo nunca deve ser salvo como picamera.py. Também deve ser observado que a visualização da câmera funciona apenas quando um monitor está conectado ao Raspberry Pi. Não funciona com acesso remoto, seja SSH ou VNC.
O método start_preview inicia uma visualização da câmera. Quando a visualização da câmera está ativa, toda a tela do monitor é ocupada pela visualização da câmera. A visualização da câmera pode ser interrompida no código Python usando o método stop_preview . Se você esqueceu de adicionar uma linha de código para interromper a visualização da câmera, ela só poderá ser interrompida saindo da execução do script. Como a tela inteira é ocupada pela visualização da câmera, pressione Ctrl+F2 para interromper a execução do código. Isso sairá com segurança da visualização da câmera.
Tirando fotos com Raspberry Pi Camera usando Python
As fotos podem ser tiradas usando o método capture da classe picamera. O método requer um caminho de arquivo para armazenar a imagem com um formato de imagem especificado corretamente. Execute o código a seguir para capturar uma imagem estática usando Python.
da importação de picamera PiCamera
do tempo importar sono
câmera = PiCâmera
câmera.start_preview
dormir (5)
camera.capture('/home/pi/Desktop/image.jpg')
câmera.stop_preview
O método sleep deve ser chamado por pelo menos 2 segundos antes de capturar a imagem. O sensor da câmera requer algum tempo para detectar os níveis de luz. Alguns segundos após iniciar a visualização da câmera dão ao módulo algum tempo para se ajustar à luz ambiente.
O código acima executa a visualização da câmera por 5 segundos. Ele tira uma foto e a salva na pasta especificada. A pasta deve existir na estrutura de diretórios do Raspberry Pi e o nome da imagem deve ser especificado em um formato reconhecido como jpg, jpeg ou png.
Para tirar várias fotos, um loop for pode ser usado da seguinte maneira.
da importação de picamera PiCamera
do tempo importar sono
câmera = PiCâmera
câmera.start_preview
para i no intervalo (5):
dormir (5)
camera.capture('/home/pi/Desktop/image%s.jpg' % i)
câmera.stop_preview
O código acima abre uma visualização da câmera, tira cinco fotos em um intervalo de 5 segundos e finalmente fecha a visualização da câmera.
Gravando vídeo com câmera Raspberry Pi usando Python
Um vídeo pode ser gravado usando os métodos start_recording e stop_recording da biblioteca picamera. O método start_recording inicia a gravação de um vídeo. Um método sleep pode ser chamado depois dele durante a duração pretendida do vídeo gravado. A gravação de vídeo pode ser interrompida usando o método stop_recording . Execute o código a seguir para gravar um vídeo usando Python.
da importação de picamera PiCamera
do tempo importar sono
câmera = PiCâmera
câmera.start_preview
camera.start_recording('/home/pi/Desktop/video.h264')
dormir (10)
câmera.stop_recording
câmera.stop_preview
O código acima grava um vídeo usando uma câmera Raspberry Pi por 10 segundos. O vídeo gravado é salvo no local especificado. O local especificado deve existir na estrutura de diretórios do Raspberry Pi. O nome do vídeo deve ser especificado no formato de arquivo adequado. A biblioteca picamera suporta formatos de vídeo h264 e mjpeg.
Girando a visualização da câmera
É possível obter uma visualização desorientada da câmera no monitor devido à instalação estranha do módulo da câmera. A visualização da câmera pode ser girada para 90˚, 180˚ e 270˚ usando a propriedade de rotação do objeto da câmera. Se a visualização da câmera estiver de cabeça para baixo, use o código a seguir.
câmera = PiCâmera
rotação da câmera = 180
Tornando a visualização da câmera transparente
É possível tornar a visualização da câmera transparente. É útil ver os erros de compilação do código enquanto a visualização da câmera está ativa. Isso é feito definindo o nível alfa da visualização da câmera. É passado como argumento para o método start_preview . O valor de um parâmetro alfa pode ser definido entre 0 e 255.
câmera = PiCâmera
câmera.start_preview(alfa=200)
Alterando o brilho da visualização da câmera
O brilho da visualização da câmera pode ser definido alterando a propriedade de brilho do objeto da câmera. O brilho pode ser definido entre 0 e 100.
câmera = PiCâmera
câmera.start_preview
câmera.brilho = 70
Alterando o contraste da visualização da câmera
O contraste da visualização da câmera pode ser definido alterando a propriedade de contraste do objeto da câmera. O contraste pode ser definido entre 0 e 100.
câmera = PiCâmera
câmera.start_preview
câmera.contraste = 70
Alterando a resolução e a taxa de quadros
Por padrão, a resolução das imagens capturadas é definida de acordo com a resolução do monitor. A resolução máxima para imagens estáticas pode ser 2596×1944 e para gravação de vídeo é 1920×1080. A resolução mínima é 64×64. A resolução pode ser alterada alterando a propriedade de resolução do objeto da câmera. Deve-se observar que se a alta resolução for selecionada, a taxa de quadros deverá ser reduzida para um funcionamento adequado, alterando a propriedade da taxa de quadros do objeto da câmera.
câmera = PiCâmera
câmera.resolução = (2592, 1944)
câmera.taxa de quadros = 15
Adicionando texto às imagens
É possível adicionar texto às imagens estáticas especificando a propriedade annotate_text do objeto câmera.
câmera = PiCâmera
câmera.start_preview
camera.annotate_text = “EEWORLDONLINE”
Alterar a fonte e a cor do texto adicionado
O tamanho da fonte, a cor e a cor de fundo do texto adicionado podem ser alterados especificando as propriedades annotate_text_size, annotate_foreground e annotate_background do objeto da câmera. Para adicionar cor ao texto, a classe de cores também precisa ser importada do picamera. O tamanho do texto pode ser definido entre 6 e 160. O tamanho do texto padrão é 32.
da importação de picamera PiCamera, Color
do tempo importar sono
câmera = PiCâmera
câmera.start_preview
câmera.annotate_text_size = 50
camera.annotate_background = Cor('azul')
camera.annotate_foreground = Cor('amarelo')
camera.annotate_text = “EEWORLDONLINE”
dormir (5)
camera.capture('/home/pi/Desktop/image.jpg')
câmera.stop_preview
Alterando a exposição das imagens capturadas
A exposição das imagens capturadas pode ser definida alterando a propriedadeposition_mode do objeto da câmera. O modo padrão é automático. Os modos de exposição suportados pela biblioteca picamera são desligado, automático, noturno, visualização noturna, luz de fundo, holofote, esportes, neve, praia, muito longo, fps fixo, anti-vibração e fogos de artifício.
câmera = PiCâmera
câmera.start_preview
camera.exposure_mode = 'praia'
dormir (5)
camera.capture('/home/pi/Desktop/beach.jpg')
câmera.stop_preview
Execute o código a seguir para percorrer todos os modos de exposição na visualização da câmera.
câmera = PiCâmera
câmera.start_preview
para exmode em camera.EXPOSURE_MODES:
câmera.exposure_mode = exmode
camera.annotate_text = “Modo de exposição: %s” % exmode
dormir (5)
câmera.stop_preview
Alterando o equilíbrio de branco da imagem
O equilíbrio de branco das imagens capturadas pode ser definido alterando a propriedade awb_mode do objeto da câmera. O modo padrão é automático. Os modos predefinidos de equilíbrio de branco suportados pela biblioteca picamera são desligado, automático, noturno, luz solar, nublado, sombra, tungstênio, fluorescente, incandescente, flash e horizonte.
câmera = PiCâmera
câmera.start_preview
camera.awb_mode = 'luz solar'
dormir (5)
camera.capture('/home/pi/Desktop/beach.jpg')
câmera.stop_preview
Execute o código a seguir para percorrer todos os modos de equilíbrio de branco na visualização da câmera.
câmera = PiCâmera
câmera.start_preview
para awbmode em camera.AWB_MODES:
Câmera. awb_mode = awbmode
camera.annotate_text = “Modo de equilíbrio de branco: %s”% awbmode
dormir (5)
câmera.stop_preview
Adicionando efeitos de imagem
A biblioteca picamera também suporta a adição de efeitos de imagem às imagens capturadas. Para adicionar um efeito de imagem, a propriedade image_effect do objeto câmera precisa ser alterada. Os efeitos de imagem suportados são nenhum, negativo, solarização, esboço, redução de ruído, relevo, pintura a óleo, hachura, gpen, pastel, aquarela, filme, desfoque, saturação, troca de cores, desbotado, posterização, colorpoint, equilíbrio de cores, desenho animado, desentrelaçamento1 e desentrelaçamento2.
câmera = PiCâmera
câmera.start_preview
camera.image_effect = 'pintura de óleo'
dormir (5)
camera.capture('/home/pi/Desktop/beach.jpg')
câmera.stop_preview
Execute o código a seguir para percorrer todos os efeitos de imagem na visualização da câmera.
câmera = PiCâmera
câmera.start_preview
para efeito na câmera.IMAGE_EFFECTS:
camera.image_effect = efeito
camera.annotate_text = “Efeito: %s” % efeito
dormir (5)
câmera.stop_preview
Conclusão
O módulo de câmera Raspberry Pi é muito divertido. A biblioteca picamera é bem escrita e poderosa. Permite tirar fotos, gravar vídeos, transmitir vídeos, transmitir vídeos para uma rede e até processar imagens com openCV. O módulo da câmera é fácil de instalar e oferece infinitas possibilidades de fazer muitas coisas interessantes.