In the previous tutorial , we successfully set up a Linux Raspberry Pi (RPi) desktop. Now let's learn Python.
We could start by explaining how to control the hardware using Python scripts, but that would defeat the purpose of using Raspberry Pi and a very high-level language (VHLL) – like Python – to control the electronics.
Of course, you can easily control electronics and design embedded applications with any microcontroller. The reason we are using RPi (a single board computer) and a VHLL is to use desktop, web and network programming capabilities in embedded applications. This allows us to design powerful and complex applications that employ user interfaces, graphics, networks and databases – to manipulate, share and use data from embedded sensors and design embedded applications that can be networked to desktop systems or servers.
With RPi, we can interface with a variety of complementary embedded devices (or circuits) that provide machine-level data in specific applications. This data can then be manipulated and used by a VHLL (like Python) to design large applications across diverse niches. This may include biometrics, robotics, automation, gaming and cloud computing, etc.
For example, we can connect a fingerprint module with Raspberry Pi and design a biometric attendance system. Using Python features, we can also develop a software solution on this biometric attendance system where an HR manager can view an attendance record, evaluate employee productivity and calculate salaries and overtime payments. We could develop fully integrated HR management software.
By simply connecting LED matrix, character LCD or graphic LCD with RPi, we can design a remotely controlled display board that displays customized messages through an RPi desktop based GUI solution. By connecting an accelerometer sensor and some buttons, we can develop a custom gamepad for gaming on Raspberry Pi.
There are limitless possibilities when you combine electronics, an operating system, and the capabilities of a VHLL. A solid foundation in Python means you can build complex applications that go beyond a basic programming hobby.
Programming language
Python is a very high-level language (VHLL) that can also be used to code at the bit and byte level. It is a general-purpose, object-oriented, cross-platform programming language that can run on all major operating systems and hardware platforms. Although Python is object-oriented, it is not limited to an OOP software design pattern.
This means that it is possible to design software solutions in Python using procedural or functional programming. Software design patterns such as Singleton, Model-View-Controller (MVC), Template, Proxy, Command, Observer, Factory and others can be implemented in Python. Python's object-oriented features are similar to C++, but simpler.
As a VHLL, Python has a high level of abstraction from the underlying hardware. Additionally, Python can be integrated with a variety of other programming languages and combined with software components written in other languages. This versatility is a software advantage in almost every niche.
However, Python is an interpreted language, so it is typically slow compared to compiled languages. This can be challenging when designing applications that need to respond to time-sensitive events. But it is possible to precompile python or use modules written in other compiled languages. And in most cases, the performance of a Python application is sufficient.
When used for applications embedded on the Raspberry Pi, users may encounter limitations. Running an embedded application with Python can be slower than running machine code on a microcontroller. The RPi also lacks some important hardware features such as analog-to-digital converters, real-time clock, and hardware interrupts.
Any requirements for analog-to-digital converters or real-time clock can be compensated by interfacing the external ADC or RTC to the RPi. For interrupts, you must rely on the Linux interrupt API. Software interrupts will need careful thread management or they will not be implemented as quickly as real hardware interrupts.
Forms
Python is a general-purpose programming language that can be used in various application domains. It has a standard library and several extension modules that allow you to use Python in various applications and software solutions.
By running Python on single-board computers like Raspberry Pi, Beaglebone and others in full Linux environments, it can be used in embedded applications. These single-board computers can be interfaced with microcontrollers and programmable chips to design interesting applications in embedded systems, robotics, automation, biometrics and other niches.
A three Python implementation, called MicroPython, can be used to program a microcontroller board such as pyBoard. This is similar to how embedded C is used to program Arduino and other microcontrollers.
MicroPython on pyBoard allows you to program microcontroller applications using Python, as well as compile Python to bytecode or machine code.
To avoid confusion, we will use conventional Python V3 on the RPi. With the support of extension modules, it will interface with electronic circuits and hardware through general purpose input/output (GPIO) and other RPi hardware interfaces. MicroPython produces executable bytecode or machine code that can be run as firmware on pyBoard.
Using RPi, we will design general-purpose Python applications that run as Linux processes rather than firmware bytecode or machine code. One advantage of using Python in statistical process control (SBC) – rather than Micropython in pyBoard – is that we get full access to Python's standard library and extension modules. Micropython lacks most of the features of the standard library.
Other advantages of using conventional Python mean that we can:
- Import hardware control modules to configure and manage electronic components through GPIO and hardware interfaces
- Use the socket library to connect to available Internet services
- Gain interpreter access to hardware devices via USB serial port and Telnet protocol
- Respond to external devices and timer events with the help of Linux APIs and special Python modules.
- Write initialization sequences that implement a Python application of any complexity.
Thanks to these features and versatility, Python has become the best choice when programming Internet of Things (IoT) applications. What's more, Python can be used for desktop programming, web development, software development, business applications, and scientific computing. With the help of Tk GUI library and toolkits such as Qt, wxWidgets, and Kivy, you can design desktop GUIs.
The Python standard library supports all major Internet protocols and the use of HTML, JSON, and XML. There are several Python frameworks for web and Internet development, including Django, Flask, Bottle, Pyramid, and Python-based content management systems – such as Django CMS and Plone.
Additionally, Python-based tools like SCons, Roundup, Apache Gump, and Buildbot can be used for software development. It can also be used for enterprise resource planning (ERP) and e-commerce solutions. There are packages available that can be used for scientific computing, data analysis, and data visualization.
Python has a lot to offer, but it is up to the developer to decide how to best use its resources with electronics.
Implementations
Python has four production-quality implementations.
1. Classic Python. This is the most up-to-date and complete implementation of Python. CPython, including its interpreter, compiler, and standard library and extension modules, is coded in the C language. It can run on any C-compatible platform. CPython is the de facto implementation of the Python programming language and is typically called “Python ”. Does not require any special programming environment.
2. Jython. Compatible with Java 7 and above, Jython is a Python implementation developed for Java Virtual Machines (JVM). The latest version, Jython 2.7.1, supports Python 2.7 but does not support Python V3. Python versions V2 and V3 are also incompatible with each other.
Jython can use all Java libraries and frameworks and is compatible with any Java class as an extension module. Jython applications are essentially pure Java applications that can run on any JVM-enabled device, available for almost all operating systems and hardware platforms.
3. FerroPython. IronPython is a .Net (Common Language Runtime or CLR) implementation of Python. CLR is now open source and ported to Linux and macOS. The CLR also has a cross-platform implementation, Mono, that can run on Windows and other non-Microsoft operating systems.
IronPython can run on any target machine with a .Net framework. It can use any CLR class as an extension module, including classes written in C# and Visual Basic .Net.
4. PyPy – PyPy. An alternative CPython implementation that can generate “just-in-time” native machine code. PyPy is faster in speed and memory management compared to all other implementations – and the credit goes to its just-in-time compilation into machine code. CPython is faster than Jython and IronPython and is only slightly behind PyPy.
PyPy is compatible with Python V2 and V3. It supports most standard Python libraries and can use any extension coded in C. By default, it is stackless and provides microthreads for concurrency.
There is also a high-performance implementation of Python known as Pyston available. However, Python implementations differ depending on the environment in which they run and the libraries, extension modules, and frameworks they can use. The selected implementation also influences the packaging and distribution of the application.
You can install and run any of the above Python implementations on Raspberry Pi. By default, the RPi uses CPython. For greater performance, PyPy can be installed, which is two to 10 times faster than conventional Python. PyPy and CPython can use extension modules coded in C, but if you need Java libraries and modules, Jython may be preferred. For CLR libraries and extension modules, consider IronPython.
Remember: the RPi was never designed for time-critical applications. Therefore, we will continue with the default installation of Python on Raspbian. It is CPython and provides all the features of the Python language.
Standard library and extension modules
The Python standard library includes built-in modules (written in C) for operating system-related tasks and modules (written in Python) for common programming tasks such as data manipulation, string manipulation, date and time operations, mathematical operations, file and directory access, data compression, etc.
Extension modules—either from the standard library or from the specific Python implementation—enable additional functionality for the underlying operating system or other software components. You can also embed Python in applications coded in other languages through application-specific Python extensions.
Some examples:
- CPython can use C++ libraries and classes as extension modules
- Jython can use Java libraries and classes as extension modules
- IronPython can use CLR libraries and classes as extension modules.
However, when using extension modules, the cross-platform portability of a Python application may be compromised and require additional dependencies. For example, Jython will require JVM to compile Java libraries and classes.
Programming environments
Typically, Python files are text files that are scripts or modules. Scripts are files that run directly as a process. Modules are source files imported to provide certain functionality to the script. Although a Python file can because modules can serve as executable scripts, providing functionality for other scripts on import.
Essentially, any Python application can be viewed as a collection of scripts and modules or just one script.
To develop software, you will need a text editor to write code for Python scripts and modules, as well as a compiler and interpreter to generate executable code. There are several integrated development environments available that combine a text editor, compiler, and interpreter into a single programming environment.
Popular integrated development environments (IDEs) for Python include IDLE, Eclipse, Geany, and Thonny. Raspbian comes with the default IDLE installation. Most IDEs provide an interpreter, through which Python codes can be executed interactively. These are called interactive sessions. These interactive sessions can be identified by a “>>>” prompt string that indicates that some Python instructions are expected to be executed.
Running scripts
Python scripts can be run in the IDE or in a command-line Python interpreter. Within the IDE, Python statements can also be executed from an interactive session, although it is typically better to wrap your application as a Python script. For example, on the RPi, Python scripts can be run in IDLE (or another Python IDE) or the Bash shell.
When running Python scripts from the shell, the “python” or “python3” command can be used. The command must follow the name and/or path of the target Python script and any optional arguments as needed. The bash shell also allows multiple lines as a single argument that can be used to execute multiple Python code statements together. However, some shells only allow a single-line command where one or more Python statements can be executed and separated by semicolons.
Python script files have a .py extension (suffix to the file name), and Python-compatible bytecode files have .pyc or .pyo extensions.
To run Python scripts from the bash shell, these commands can be used:
Python.py script name
or
python3 script-name.py
Python 2.7 can be the default version of Python on a machine. When running Python command, the script will be executed by default version. Running python3 or python3.5 command, the script will be explicitly executed by versions V3 or V3.5 respectively (if installed).
On Raspbian, Python scripts can be written and compiled using IDLE. You can open IDLE by navigating to Programming -> Python 3 (IDLE).
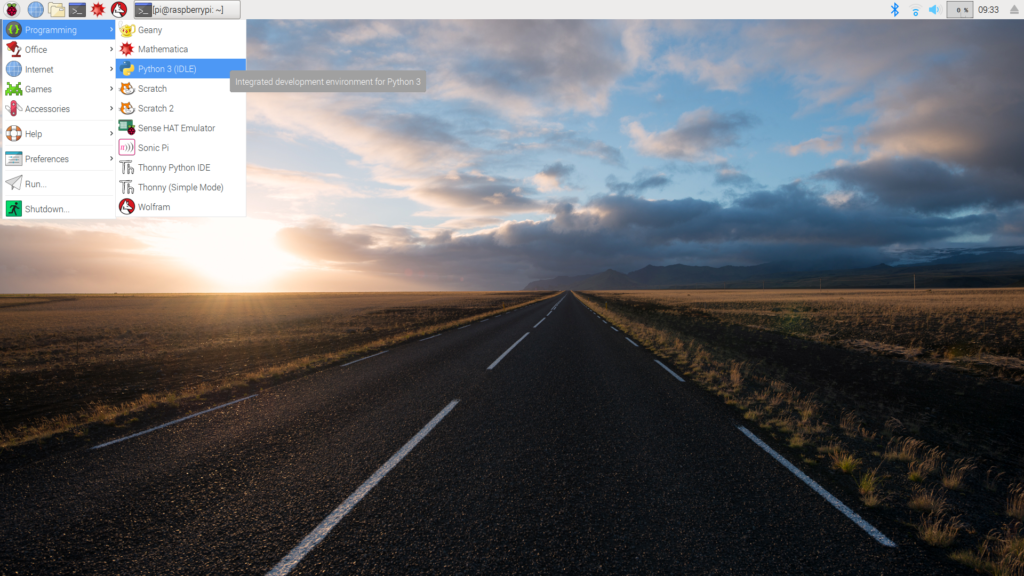
Navigating to IDLE (Python 3) on Raspbian.
IDLE will open with an active, interactive session like this:
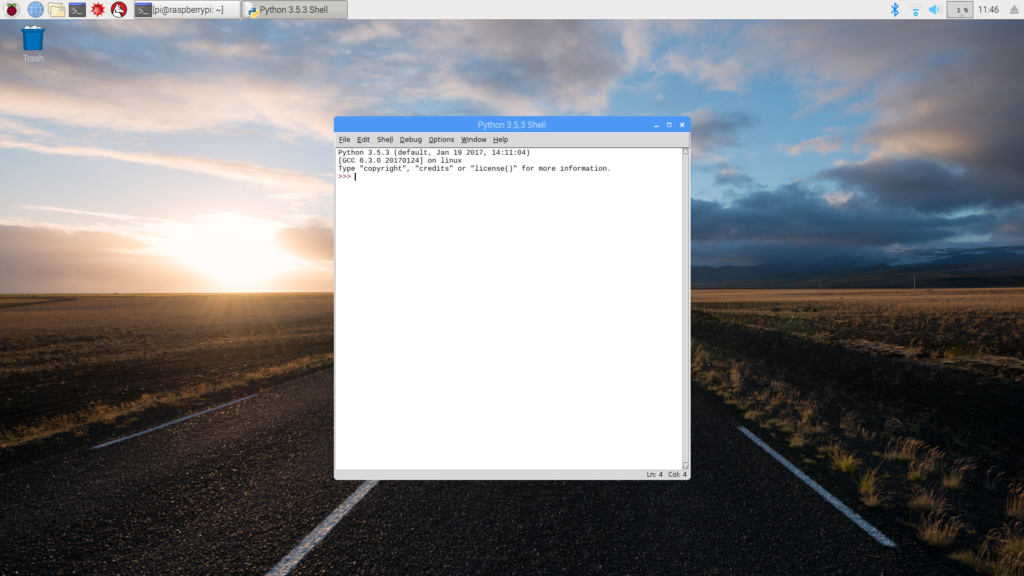
The IDLE Python IDE on Raspbian.
To write a Python script or module, click Files -> New File.
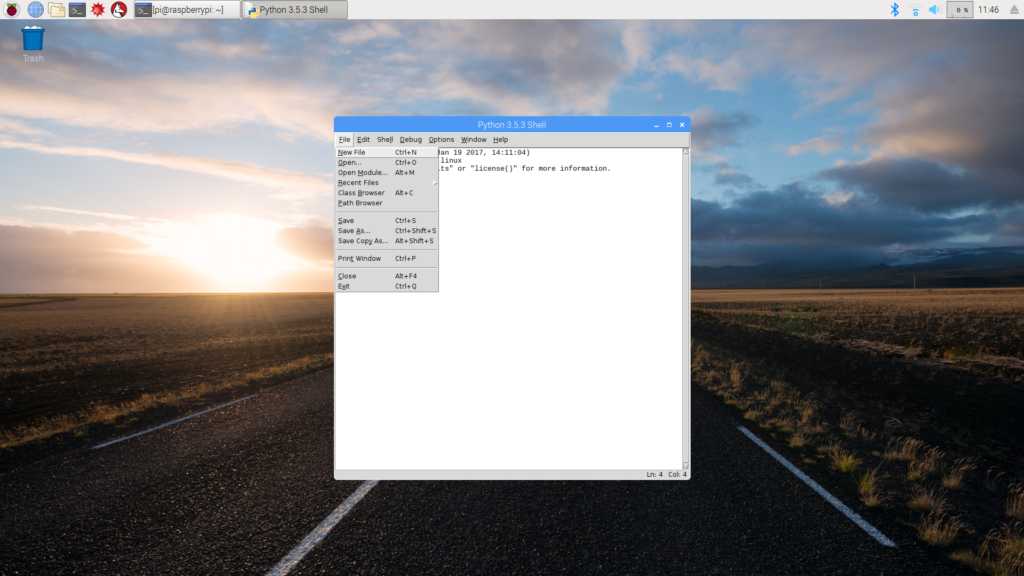
Creating a new file to write Python script in IDLE on Raspbian.
Write your Python script in the text editor and save it by clicking Files -> Save.
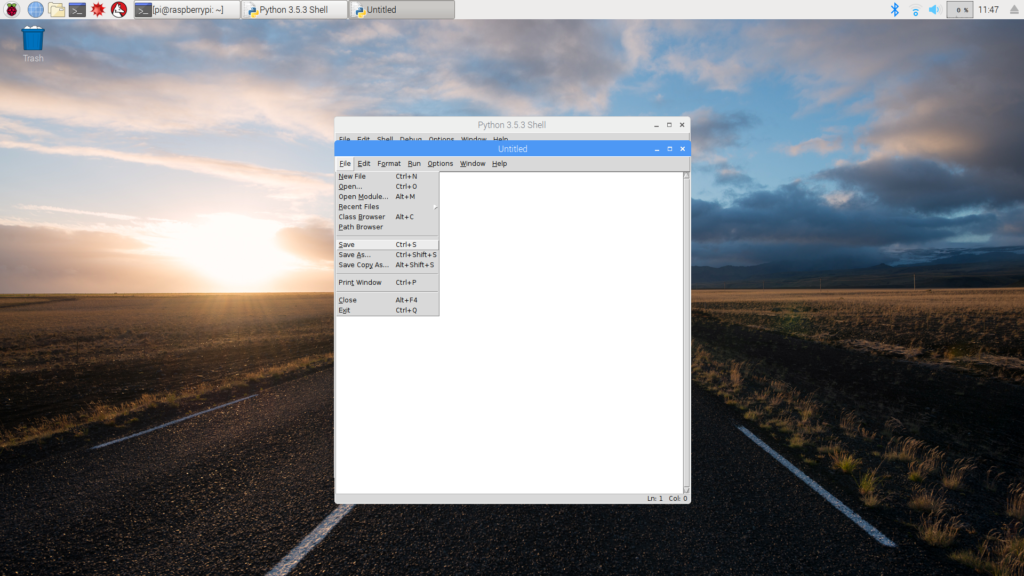
Saving a Python script to IDLE on Raspbian.
To run a Python script, open it in the IDE and click Run -> Run Module or press F5.
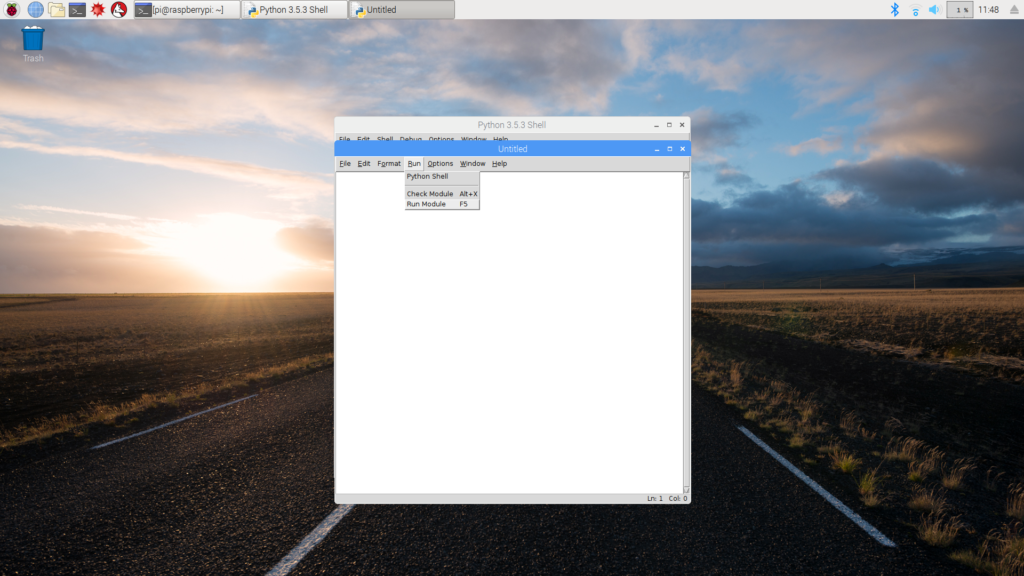
Running Python script in IDLE on Raspbian.
Resources
Python is a mature programming language that offers continuous learning. The best place to explore Python is on its website . The same happens with Jython , FerroPython and PyPy . Standard library packages and extension modules can be downloaded from the Python Package Index .
Costs
CPython is covered by the Python Software Foundation Version 2 License, which is compatible with the GNU Public License (GPL). Jython, IronPython and PyPy are also covered by free licenses. This means that anything downloaded from the main Python, Jython, IronPython, and PyPy sites is completely free.
Many third-party sources, tools, and Python extension modules offer similar licenses or are covered by the GPL or the Lesser GPL (LGPL). Some commercially developed modules and tools may require a fee. However, Python can be used for any proprietary, free, or open-source software development.
In the next tutorial we will cover the fundamentals of the Python programming language.