In this article we will be controlling devices that do not support WhatsApp, but support other communication protocols such as MQTT, TCP, IMAP, etc. If a home is installed with home automation devices that do not support Whatsapp, we will communicate with these devices (controllers) using protocols supported by these devices.
This way, we will be able to control the household appliances connected to these devices.
Required components
Required tools/required libraries
Python-based WhatsApp API – yowsup
MQTT library – paho
Python ID
Arduino IDE
Technical information
The protocol bridge can also control devices that do not support the WhatsApp API. This means that we will simply send data from one protocol to another. This article will demonstrate MQTT and WhatsApp Bridge to control devices.
Block diagram
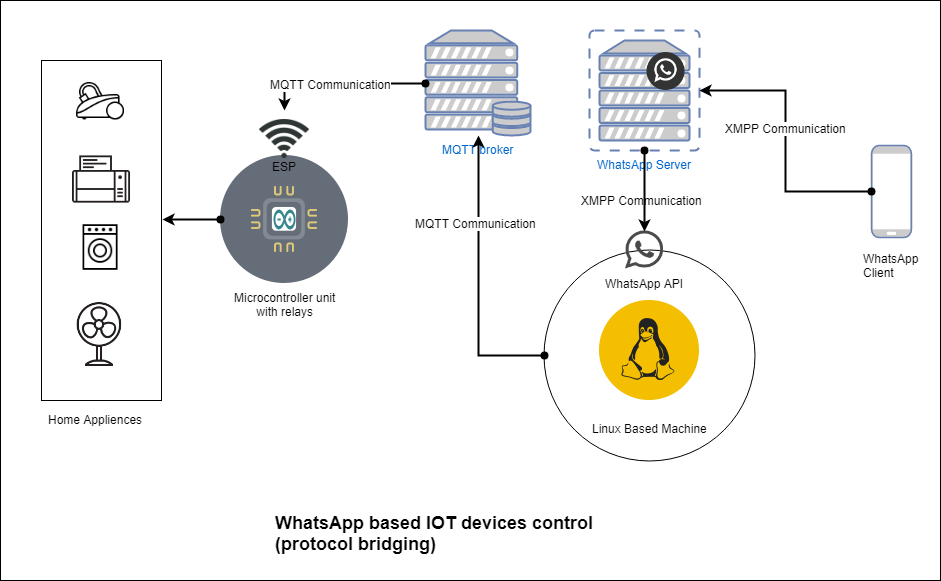
Figure 1 Bridge between WhatsApp and MQTT protocols
All communication will be through a Python script, which is installed on a Linux-based system. The Python script will have WhatsApp API and MQTT library to communicate with both protocols.
The script can send and receive messages on WhatsApp and MQTT.
A microcontroller (ATmega 328p) is connected to the home appliances through relay circuits. For communication purposes, ESP is connected to the microcontroller.
ESP is installed with code that receives messages on a specific topic and sends commands to the microcontroller through serial UART.
So, now when a user sends a message on WhatsApp, it goes to the microcontroller through our Python script.
Circuit Diagram
This board is connected to a light switch with a relay circuit. We can also use the Arduino Uno board instead of the custom 328 board.
How the system works
When a user sends a message to our Linux system on WhatsApp, the script reads the message. The IoT device, which supports the MQTT protocol, listens for messages on a specific topic. These messages command the device to turn ON and OFF a device.
So now messages read by the Python script are scanned for commands if found. Specific commands are sent to the device in the MQTT protocol. When the device reads these commands, it acts on them by turning the HIGH (ON), LOW (OFF) pins
Understanding the source code
We have two types of source code, one for Arduino + ESP and another for Python script installed on Linux.
Code for Arduino
The Arduino is installed with a code that receives data in series. When a specific string is received as “ON”, the relay pin will be ON (HIGH) and on receiving “OFF”, the relay will be turned OFF.
if (rec == “ON”) {
digitalWrite(relay, HIGH);
Serial.print(“Relay on”);
rec = “”;
}
if (rec == “OFF”) {
digitalWrite(relay, LOW);
Serial.print(“Relay off”);
rec = “”;
}
Code for ESP
The ESP is connected to the Arduino on a serial port and also subscribed to an MQTT topic to receive data from it. Basically, it sends the data received on the MQTT topic to the serial port and the data from the serial to the MQTT topic.
To learn more about ESP and MQTT, see our previous articles.
Code for Python script
The Python script is installed with the WhatsApp “Yowsup” API to read and send WhatsApp messages. There are two files in this script run.py and layer.py .
Understanding the Run.py File
We will call our libraries at the top of the file
from yowsup.stacks import YowStackBuilder
from yowsup.layers.auth import AuthError
from yowsup.layers import YowLayerEvent
from yowsup.layers.network import YowNetworkLayer
from yowsup.env import YowsupEnv
We will also attach the layer file at the top because the main class “Ecolayer” exists inside this file.
from EchoLayer layer import
We can name any layer file, but we have to put the same name here.
Inside py, we will declare our main variable for password and events we want to occur.
credentials = (“91xxxxxxxxxx”, “HkhWVW5/Wnr493HXK8NKl/htpno=”)
Now we move to the layer and build the stack. Additionally, the loop that will keep the connection alive is called.
stack.setCredentials(credentials)
stack.broadcastEvent(YowLayerEvent(YowNetworkLayer.EVENT_STATE_CONNECT)) #sending the connection signal
stack.loop #this is the mainloop program
Understanding the layer.py file
This file contains the protocol library for MQTT and is capable of receiving WhatsApp messages.
Understanding how messages are received from WhatsApp
This file contains the class that will receive all incoming messages for this number, and which will be a callback entity so that any other loops can be executed within the file.
@ProtocolEntityCallback(“message”)
def onMessage(self, messageProtocolEntity):
if it's true:
The message data and the form of the number the message came from can be obtained below.
incomming_message_data = messageProtocolEntity.getBody
This will get the message body, which is the actual message. It will be stored in a string variable “incomming_message_data”
incomming_message_sender = messageProtocolEntity.getFrom
This line will store the contact number of the message received in the string variable “incomming_message_sender”
Understanding MQTT layers for sending and receiving
Firstly, we will import the necessary libraries for MQTT.
import paho.mqtt.client as MQTT
import paho.mqtt.publish how to publish
Now we will declare a variable called client with mqtt client.
client = mqtt.Cliente
Now we will make two function callbacks 1. To receive messages, 2. Do something on successful connection.
client.on_connect=on_connect
client.on_message=on_message
Finally, we will connect to the MQTT broker on a port and start the client inside a non-blocking loop
client.connect(“corretor.hivemq.com”, 1883, 60)
client.loop_start
After the connection is successful, we can send messages using this
publish.single(topic, message_data, hostname=”broker.hivemq.com”)
When any message is received on WhatsApp, it is stored in a string, and then that string is scanned for some keywords that define that message as a command to turn on/off the light.
elif(“lights on” in incomming_msg): #Do something in the match
If the condition is met, we send the control command to the MQTT broker.
publish.single(“ts/light”, “ON”, hostname=”broker.hivemq.com”)
When any unrecognized message is received, the message on WhatsApp responds that it is invalid.
And this is how we can use the protocol bridge to control devices with WhatsApp.