In this article, we will learn how to control our IoT home appliances using the WhatsApp messaging app to communicate easily and effectively with these devices.
Therefore, we will send WhatsApp messages to various “lights on” and the lights in our house will be turned ON.
Required components
Required tools/required libraries:
- WhatsApp API Library – Yowsup
- Python-based serial communication library – pyserial
- Linux-based machine
- Arduino IDE
Technical information
To control anything on the internet, we will need a protocol. WhatsApp works on XMPP (Extensible Messaging and Presence Protocol). We will be using their API to send and receive messages. The messages received will act as commands for a specific task, such as turning on/off the light.
Block diagram
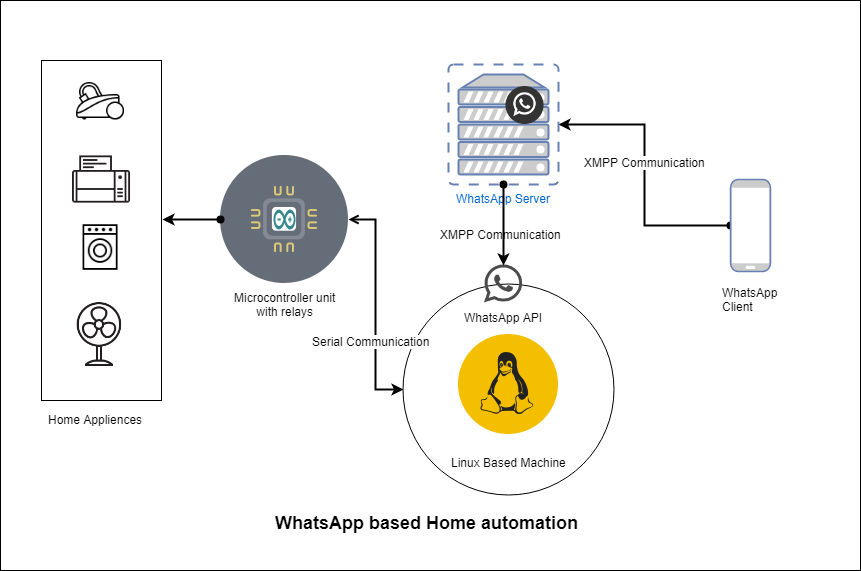
Figure 1 WhatsApp-based home automation
- Home appliances are connected through a relay to a microcontroller that controls them when a specific ON/OFF command is received through serial communication.
- The microcontroller (Arduino UNO 3 board) is connected to a Linux-based system via serial communication.
- A WhatsApp API (Yowsup) is installed on the system with a phone number. Which can send and receive WhatsApp messages.
Circuit Diagram
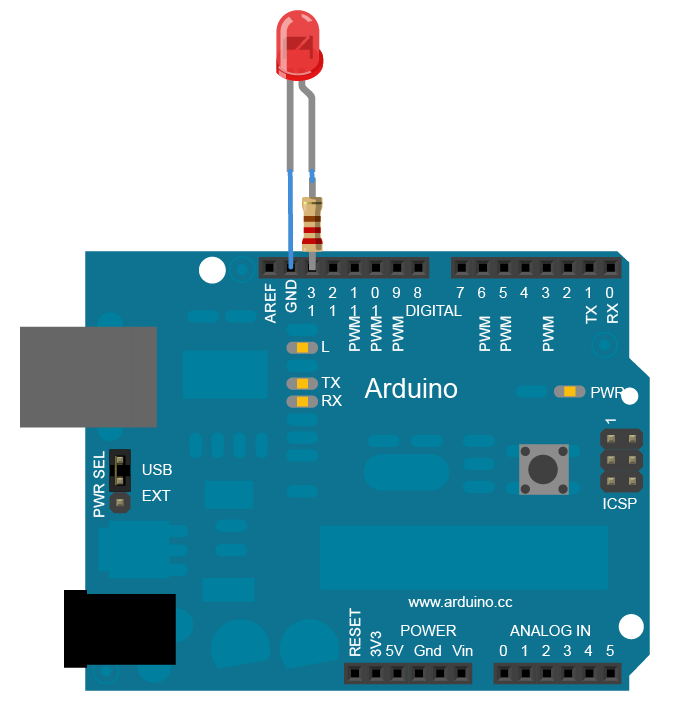
Figure 2 LED connected to Arduino Uno
How does the system work?
- When someone sends a message to the number where WhatsApp is installed on the Linux system.
- The message is received into the system via a Python script executing a message receiving script and parsed for commands such as “turn lights on/off”.
- When any command matches the predefined commands, the script sends that command to the microcontroller connected to the serial communication port.
- If the “turn on lights” command is received in a message, the script understands that it is to turn on the light, so it will send the “ON” command to the microcontroller through serial communication. The controller will turn on the light by turning on the relay.
IoT devices do not directly support the WhatsApp API, so data must be transferred from one protocol to another. In the next article, “Protocol Bridge”, we will see how to redirect messages sent from WhatsApp to MQTT.
Installing the WhatsApp API on Linux
Step 1 – Preparing the system
This API works in python, so we need to first configure the environment to install it on the system. We can do this by typing the following command in the terminal.
sudo apt-get update
sudo apt-get install python-dateutil
sudo apt-get install python-setuptools
sudo apt-get install python-dev
sudo apt-get install libevent-dev
sudo apt-get install ncurses-dev
Step 2 – Downloading the API
Enter the following command in the terminal.
git clone git://github.com/tgalal/yowsup.git
Now go to youwsup folder and type the following to install it on the system
sudo python setup.py install
Now that we have installed this in our system it is time to register the WhatsApp number.
Note- Only one number per device is allowed on WhatsApp, so you need a new number to install WhatsApp on Linux.
Step 3 – Configuring the API
- The Yowsup API comes with a command-line utility used for registration.
- To register the phone number, we need to download the latest WhatsApp APK and do the following things.
- We need to download the latest version of WhatsApp, calculate some MD5 hash and write it to a file in WhatsApp API. This way, WhatsApp doesn't crash and ask for an update.
- To do this, follow the steps.
- Download this file –py
- Download WhatsApp – apk
- Put them in a folder and type the following command
python dexMD5.py WhatsApp.apk
- You will see the following output
Version: 2.17.344
ClassesDex: OxVSHnBDYNBZmsgagatF9+A==
- Now write the Version and ClassessDex values to a file inside the API location
yowsup/env/env_android.py
- Now type the following command to reinstall the API
python setup.py compilation
python setup.py installation
Step 4. – Registering the user
4.1 – Obtaining the verification code
Now comes the registration on the WhatsApp server, which can be done through the “yowsup-cli” command line utility, using the following command.
python registry yowsup-cli –requestcode sms –phone 91xxxxxxxxxx –cc 91 –mcc 222 –mnc 10 -E android
Enter your phone number at 91XXXXXXXXX
The MCC and MNC codes can be found at this link –
After entering the following command, you will receive a message with a 6-digit verification code. Keep this number stored. It will be used in the next step.
4.2 – Obtaining password for login
To log in to WhatsApp, we will need a password string, a base64 string. We can get this by replacing xxx-xxx with the six-digit number.
register python yowsup-cli –register xxx-xxx –phone 91xxxxxxxxxx –cc 91 -E android
After that you will see output like this
status: ok
type: free
pw: xxxxxxxxxxxxxxxxxx= #this is the password
price: ₹55
expiration_price: 1509040085
currency: INR
cost: 55.00
validity: 4444444444.0
login: 91xxxxxxxxxx
type: new
With this installation, we get two things: a phone number on which our API is installed and a password for login. Keep them stored.
Note – Phone number must be used with country code
Telephone – 91XXXXXXX
Password – Gbuioa254ui25j2=XXXXX
Developing the source code
To develop the code for automation, we have to see how the API can be called. Furthermore, we need to write a small script on Arduino to control the relay/led. There may be steps to follow to understand how the source code works.
Linux system code
On this side we will implement these resources.
- Understanding the API architecture
- Calling the API
- Controlling the devices
Understanding API Architecture
Yowsup python application works on stacks of layers. Data can be transferred from layer to layer.
If we want to send a message, we will send that message to the lower layer.
self.toLower(outgoingMessageProtocolEntity)
If you want to receive messages, they will be accessed from the upper layer, which will be the current layer of the stack.
Calling the API
To call the API we will be making two python scripts. One will be to pass objects like username and passwords onto the stack and start pushing. The second will be our main message receiving layer, where we will receive messages and perform tasks.
So the two files will be run.py and layer.py
Understanding the Run.py File
- We will call our libraries at the top of the file
from yowsup.stacks import YowStackBuilder
from yowsup.layers.auth import AuthError
from yowsup.layers import YowLayerEvent
from yowsup.layers.network import YowNetworkLayer
from yowsup.env import YowsupEnv
- We will also attach the layer file at the top because the main class “Ecolayer” exists inside this file.
from EchoLayer layer import
- We can name any layer file, but we have to put the same name here.
- Inside py, we will declare our main variable for password and events we want to occur.
credentials = (“91xxxxxxxxxx”, “HkhWVW5/Wnr493HXK8NKl/htpno=”)
- Now we move to the layer and build the stack. Additionally, the loop that will keep the connection alive is called.
stack.setCredentials(credentials) stack.broadcastEvent(YowLayerEvent(YowNetworkLayer.EVENT_STATE_CONNECT)) #sending the connection signal
stack.loop #this is the mainloop program
Understanding the layer.py file
This file contains the class that will receive all incoming messages for this number, and which will be a callback entity so that any other loops can be executed within the file.
@ProtocolEntityCallback(“message”)
def onMessage(self, messageProtocolEntity):
if it's true:
The message data and the number the message arrived from can be obtained using the following.
incomming_message_data = messageProtocolEntity.getBody
This will get the message body, which is the actual message. It will be stored in a string variable “incomming_message_data”
incomming_message_sender = messageProtocolEntity.getFrom
This line will store the contact number of the message received in the string variable “incomming_message_sender”
Using these two values, we can do anything: receive a specific message or from a specific number. This function will always continue to receive data as it arrives.
Below is how we will use them to carry out our home automation project.
Controlling the devices
To control home appliances, we need to write code that can interact with the code written on Arduino.
We will use this simple approach in which we will write a code on Arduino that can control the output of a pin when such commands are received.
Now we open the serial port for communication, so in the code we will import our “pyserial” library.
import series
ser = serial.Serial(port, baud, timeout = 1)
To turn on or off any pin in Arduino, we need to send data in series. Also, first we need to read the received WhatsApp message.
elif(“lights on” in incomming_msg):
be.write(“on”.encode('ascii'))
This line says if there are “lights on” in the “incoming_msg” string (which is the message we received), then send the “on” string on the serial port.
The rest can be understood within the Arduino code.
To send the message back to WhatsApp, we will use this line,
outgoingMessageProtocolEntity = TextMessageProtocolEntity(message_send,to = sender_jid)
Hardware Code (Arduino)
On the Arduino, we will simply write code that listens for input strings on the serial port, and if it says “on” we will set a pin that the LED is connected to HIGH, or if it says “off” we will turn the pin on. LED pin LOW.
If(Serial.available ){
String data = Serial.readString;
if(data == “enabled”){
digitalWrite(13,HIGH);
}
Otherwise, if(data == “off”){
digitalWrite(13,DOWN);
}
}
This is how our automation code works. To learn more about XMPP read our previous articles.
Observation:
- Do not use WhatsApp API (Yowsup) with Raspbian operating system, or your number will be banned from using WhatsApp. Install it on Ubuntu or any other Linux distribution.
- WhatsApp from a number can only be installed on one device at a time.
- The frequency of sending messages should not be fast, as 30 messages per minute is the limit you can exceed, above that there is a chance that your number will be blocked on WhatsApp.