In this project, we will design a simple application using a 3.5 inch TFT LCD that displays different types of graphics and text messages with Arduino.
TFT LCDs are the most popular color displays – the displays on smartphones, tablets and laptops are actually just TFT LCDs. There are TFT LCD shields available for Arduino in a variety of sizes, such as 1.44″, 1.8″, 2.0″, 2.4″ and 2.8″. The Arduino is a pretty humble machine when it comes to processing or controlling graphics. After all, it is a microcontroller platform, and graphics applications generally require much greater processing resources. Still, Arduino is capable enough to control small display units. TFT LCDs are full-color display screens that can host beautiful user interfaces.
Most smaller TFT LCD shields can be controlled using the Adafruit TFT LCD library. There is also a larger 3.5-inch TFT LCD screen, with an 8-bit ILI9486 driver.
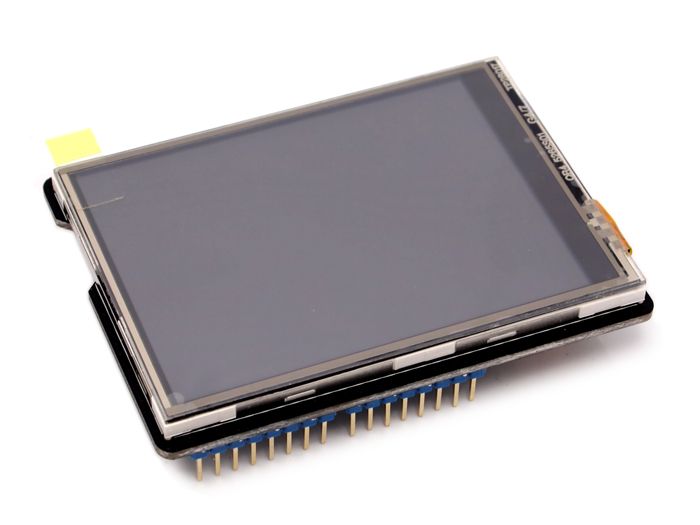
MCUFRIEND TFT LCD Touch Screen Shield
The Adafruit library does not support the ILI9486 driver. In fact, the Adafruit library is written to only control TFT displays smaller than 3.5 inches. To control the 3.5 inch TFT LCD touch screen, we need another library. This is MCUFRIEND_kbv. The MCUFRIEND_kbv library is, in fact, even easier to use compared to the Adafruit TFT LCD library. This library only requires the instantiation of a TFT object and does not even require pin connections to be specified.
The 3.5-inch TFT LCD touch screen is almost the size of the Arduino UNO, and on top of the Arduino board, the shield looks really glamorous.
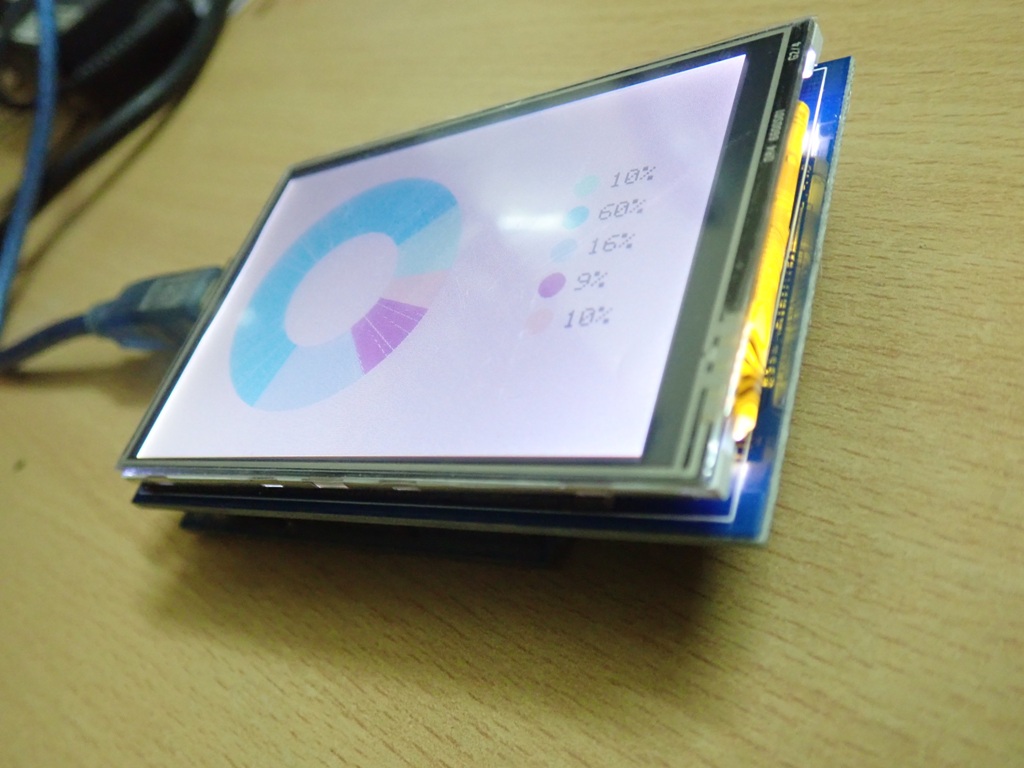
MCUFRIEND TFT LCD Touchscreen Shield on Arduino UNO
The 3.5 inch TFT LCD can be used for a number of applications like –
- To display text and fonts.
- To display interactive graphs and figures.
- To display bitmap images.
- To display RGB color photos and images.
- To use as a touch screen.
- To host graphical interfaces with touch input.
- To access digital signatures.
- To host video games with touch input.
- To host touch input apps like Paint and Calculator.
What do you need?
- Arduino UNO/Mega2560/Leonardo/Due/Zero/MO-Pro x1
- 3.5 inch TFT LCD touch screen shell
TFT LCDs for Arduino
User interfaces are an essential part of any embedded application. The user interface allows any interaction with the end user and makes the final use of the device possible. User interfaces are hosted using a series of devices such as seven-segment, character LCDs, graphical LCDs, and color TFT LCDs. Of all these devices, only color TFT displays are capable of hosting sophisticated interfaces. A sophisticated user interface may have many data fields to display, or it may need to host menus and submenus or host interactive graphics. A TFT LCD is an active matrix LCD capable of hosting high-quality images.
Arduino operates at low frequency. This is why it is not possible to render high definition images or videos with Arduino. However, the Arduino can control a small TFT screen that renders graphically enriched data and commands. By interfacing a TFT LCD touch screen with Arduino, you can render interactive graphics, menus, tables, graphs, and user panels.
Some of the popular color TFT LCDs available for Arduino include 3.5″ 480×320 display, 2.8″ 400×200 display, 2.4″ 320×240 display, and 1.8″ 220×176 display. A TFT screen of appropriate size and resolution can be selected according to a certain application.
If the user interface has only graphical data and commands, Arduino Atmega328 boards can control the display. If the user interface is a large program that hosts multiple menus and/or submenus, the Arduino Mega2560 should be preferred to control the TFT display. If the user interface needs to host high-resolution images and motion, Arduino ARM core boards like DUE should be used to control the TFT display.
MCUFRIEND_kbv Library
The Adafruit TFT LCD library only supports small TFT displays. For large TFT screens such as 3.5-inch, 3.6-inch, 3.95-inch TFT LCDs, including 2.4-inch and 2.8-inch TFT LCDs, the MCUFRIEND_kbv library is useful. This library is designed to control 28-pin TFT LCD shields for Arduino UNO. Also works with Arduino Mega2560. In addition to UNO and Mega2560, the library also supports LEONARDO, DUE, ZERO and M0-PRO. It also works on NUCLEO-F103 and TEENSY3.2 with Sparkfun adapter. Mcufriend style shields tend to have a resistive TouchScreen at A1, 7, A2, 6, but they are not always in the same direction of rotation. The MCUFRIEND_kbv library can be included in an Arduino sketch in the library manager.
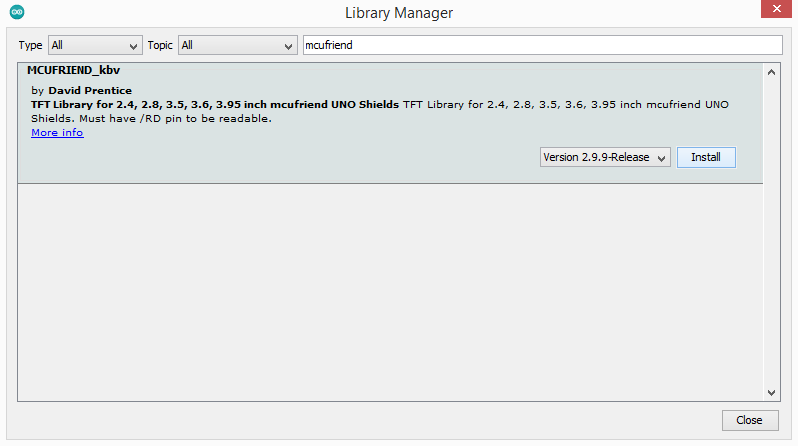
MCUFRIEND TFT LCD Touchscreen Shield Library
The sketch will also need the Adafruit_GFX library to draw text and graphics on the TFT display.
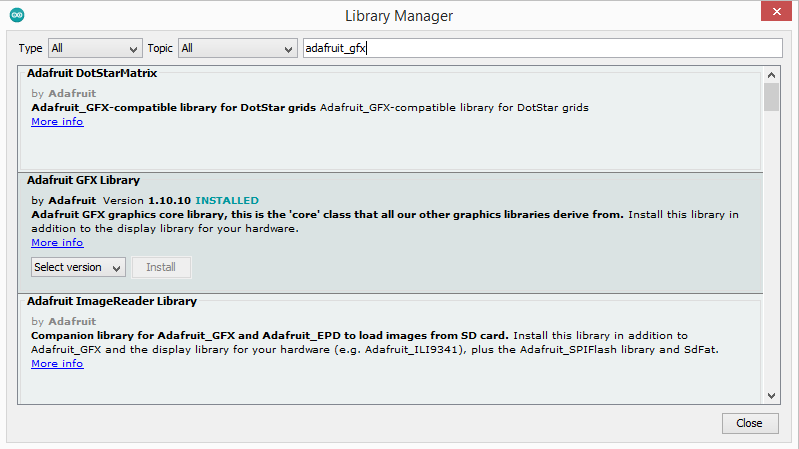
Adafruit GFX library for small TFT LCDs
Circuit Connections
The 3.5 inch TFT LCD shield needs to be connected to the Arduino board. Mcufriend style shields are designed to fit all Arduino boards mentioned above. The shields have a TFT touchscreen that can display full-color images and interfaces, and a micro SD card reader for saving images and other data. A 3.5 inch TFT LCD touch screen has the following pin diagram.
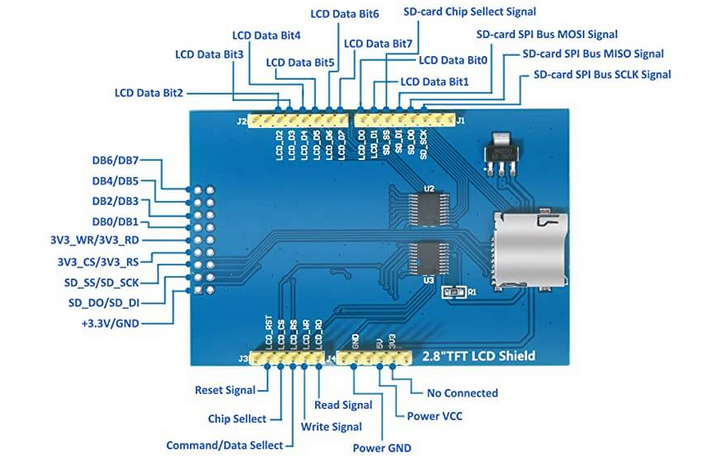
MCUFRIEND TFT LCD Touch Screen Shield Pin Diagram
Programming Guide
In the Arduino sketch, the following libraries essentially need to be included.
#include “Adafruit_GFX.h”#include “MCUFRIEND_kbv.h”
The following libraries can be included depending on your application requirements.
#include “TouchScreen.h” // only when you want to use the touch screen
#include “bitmap_mono.h” // when you want to display a bitmap image from the library
#include “bitmap_RGB.h” // when you want to display a bitmap image from the library
#include “Fonts/FreeSans9pt7b.h” // when you want other fonts
#include “Fonts/FreeSans12pt7b.h” // when you want other fonts
#include “Fonts/FreeSerif12pt7b.h” // when you want other fonts
#include “FreeDefaultFonts.h” // when you want other fonts
#include “SPI.h” // using sdcard to display bitmap image
#include “SD.h”
Firstly, an object of class MCUFRIEND_kbv needs to be instantiated to provide SPI communication between LCD and Arduino.
MCUFRIEND_kbv tft;
To start the TFT LCD, its ID needs to be read and provided to the Begin method.
uint16_t ID = tft.readID;
tft.begin(ID);
The TFT screen resolution can be determined using the following code.
screen_width = tft.width; //int16_t width(void);
Serial.print(“TFT LCD screen width: “);
Serial.println(screen_width); screen_height = tft. height ; //int16_t height(void);
Serial.print(“TFT LCD screen height: “);
Serial.println(screen_height);
The screen color can be set using the following method.
tft.fillScreen
This method can take any of the following arguments.
#define BLACK 0x0000
#define NAVY 0x000F
#define DARK GREEN 0x03E0
#define BROWN 0x7800
#define PURPLE 0x780F
#define OLIVEIRA 0x7BE0
#define LIGHT GRAY 0xC618
#define DARKGREY 0x7BEF
#define BLUE 0x001F
#define GREEN 0x07E0
#define CYAN 0x07FF
#define RED 0xF800
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
#define ORANGE 0xFD20
#define GREEN YELLOW 0xAFE5
#define PINK 0xF81F
The following method is used to set the cursor position on the TFT LCD.
tft.setCursor(x,y); //setCursor(int16_t x, int16_t y)
The following methods are used to set the text color.
tft.setTextColor
tft.setTextColor(t,b); //setTextColor(uint16_t t, uint16_t b)
The following method is used to set the text size.
tft.setTextSize(s); //setTextSize(uint8_t s)
The following methods are used to display text on TFT LCD.
tft.write(c); //write(uint8_t c)
tft.println(“www.Electropeak.com”);
tft.print(“www.Electropeak.com”);
The drawPixel function fills a pixel at location x and y with color t. The readPixel function reads the color of a pixel at location x and y.
tft.drawPixel(x,y,t); //drawPixel(int16_t x, int16_t y, uint16_t t)
tft.readPixel(x,y); //uint16_t readPixel(int16_t x, int16_t y)
The drawFastVLine function draws a vertical line that starts at x, y location, and its length is h pixel and its color is t. The drawFastHLine function draws a horizontal line that starts at location x and y, and the length is w pixel and the color is t. The drawLine function draws a line that starts at xi and yi, locationends is at xj and yj, and the color is t. These methods draw lines that are 5 pixels thick.
tft.desenharVLineFast(x,y,h,t);
//drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t t)tft.drawFastHLine(x,y,w,t);
//drawLineFastHLine(int16_t x, int16_t y, int16_t w, uint16_t t)tft.drawLine(xi,yi,xj,yj,t);
//drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t t)
The fillRect function draws a filled rectangle at locations x and y. w is the width, h is the height, and t is the color of the rectangle. The drawRect function draws a rectangle at locations x and y with width w, height h and color t. The fillRoundRect function draws a rectangle filled with radii r rounded corners at location x and y and width w and height h and color t. The drawRoundRect function draws a rectangle with radius r rounded corners at location x and y and width w and height h and color t.
tft.fillRect(x,y,w,h,t);
//fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t t)tft.drawRect(x,y,w,h,t);
//drawRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t t)tft.fillRoundRect(x,y,w,h,r,t);
//fillRoundRect (int16_t x, int16_t y, int16_t w, int16_t h, uint8_t R , uint16_t t)tft.drawRoundRect(x,y,w,h,r,t);
//drawRoundRectangle(int16_t x, int16_t y, int16_t w, int16_t h, uint8_t R , uint16_t t)
The drawCircle function draws a circle at x and y location and r radius and color. The fillCircle function draws a filled circle at x and y location and r radius and color.
tft.drawCircle(x,y,r,t);
//drawCircle(int16_t x, int16_t y, int16_t r, uint16_t t)tft.fillCircle(x,y,r,t);
//fillCircle(int16_t x, int16_t y, int16_t r, uint16_t t)
The drawTriangle function draws a triangle with three corner locations x, y, z and colors t. The fillTriangle function draws a triangle filled with three corner locations x, y, z, and t colors.
tft.drawTriangle(x1,y1,x2,y2,x3,y3,t);
//drawTriangle(int16_t x1, int16_t y1, int16_t x2, int16_t y2, int16_t x3, int16_t y3, // uint16_t t)tft.fillTriangle(x1,y1,x2,y2,x3,y3,t);
//fillTriangle(int16_t x1, int16_t y1, int16_t x2, int16_t y2, int16_t x3, int16_t y3, // uint16_t t)
The following function rotates the screen by a certain angle.
tft.setRotation(r); //defineRotation(uint8_t r)
The following function inverts the screen colors.
tft.invertDisplay(i); //invertDisplay(boolean i)
The following function gives the RGB code and gets the UTFT color code.
tft.color565(r,g,b); //uint16_t cor565(uint8_t r, uint8_t g, uint8_t b)
The following function scrolls the screen. Maxroll is the maximum height of your roll.
for (uint16_t i = 0; i < maxscroll; i++) {
tft.vertScroll(0, maxscroll, i);
delay(10);}
The following function resets the screen.
tft.reset;
Arduino Sketch
How the project works
The code fills a rectangle and then draws a rectangle within which the text “EEWORLDONLINE” is displayed. Then lines, circles, rectangles and squares are drawn on the screen. The project ends with a greeting and a message.
Results
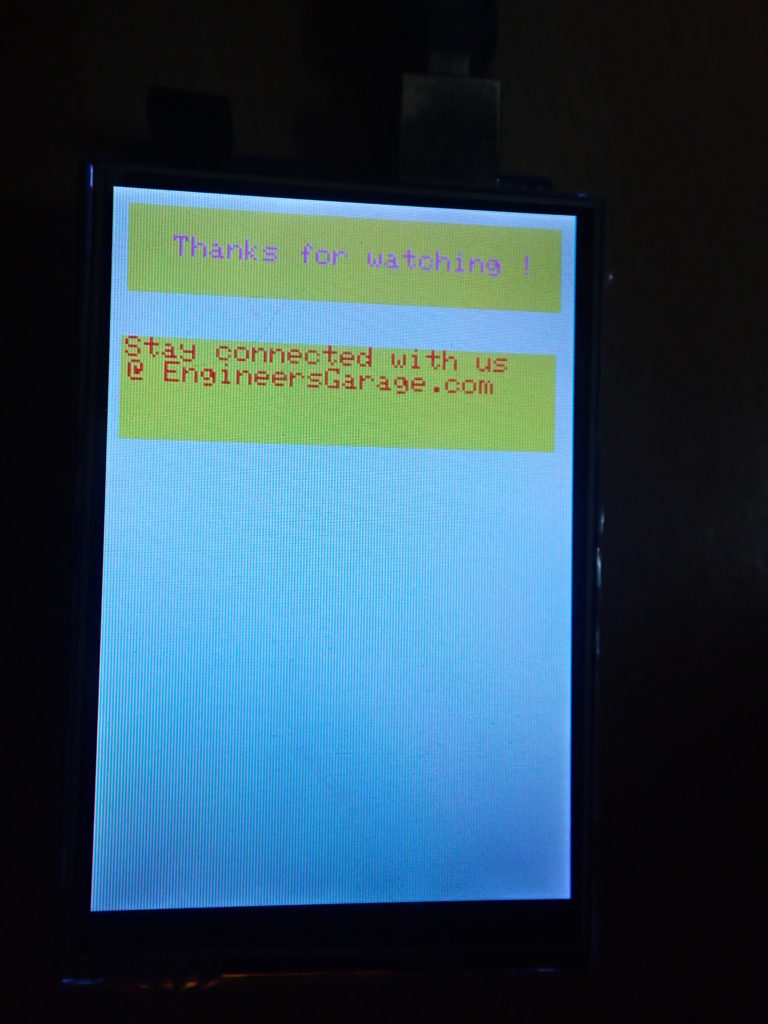
MCUFRIEND TFT LCD Touchscreen Shield with Arduino UNO